By: CS2103-AY1819S2-W15-2
Since: Feb 2019
- 1. Setting up
- 2. Design
- 3. Implementation
- 4. Documentation
- 5. Testing
- 6. Dev Ops
- Appendix A: Suggested Programming Tasks to Get Started
- Appendix B: Product Scope
- Appendix C: User Stories
- Appendix D: Use Cases
- Appendix E: Non Functional Requirements
- Appendix F: Glossary
- Appendix G: Instructions for Manual Testing
1. Setting up
1.1. Prerequisites
-
JDK
9
or laterJDK 10
on Windows will fail to run tests in headless mode due to a JavaFX bug. Windows developers are highly recommended to use JDK9
. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
1.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests. -
Open
MainWindow.java
and check for any code errors-
Due to an ongoing issue with some of the newer versions of IntelliJ, code errors may be detected even if the project can be built and run successfully
-
To resolve this, place your cursor over any of the code section highlighted in red. Press ALT+ENTER, and select
Add '--add-modules=…' to module compiler options
for each error
-
-
Repeat this for the test folder as well (e.g. check
HelpWindowTest.java
for code errors, and if so, resolve it the same way)
1.3. Verifying the setup
-
Run the
seedu.address.MainApp
and try a few commands -
Run the tests to ensure they all pass.
1.4. Configurations to do before writing code
1.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
1.4.2. Updating documentation to match your fork
After forking the repo, the documentation will still refer to the CS2103-AY1819S2-W15-2/main
repo.
If you plan to develop this fork as a separate product (i.e. instead of contributing to CS2103-AY1819S2-W15-2/main
), you should do the following:
-
Configure the site-wide documentation settings in
build.gradle
, such as thesite-name
, to suit your own project. -
Replace the URL in the attribute
repoURL
inDeveloperGuide.adoc
andUserGuide.adoc
with the URL of your fork.
1.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
1.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading Section 2.1, “Architecture”.
-
Take a look at Appendix A, Suggested Programming Tasks to Get Started.
2. Design
2.1. Architecture
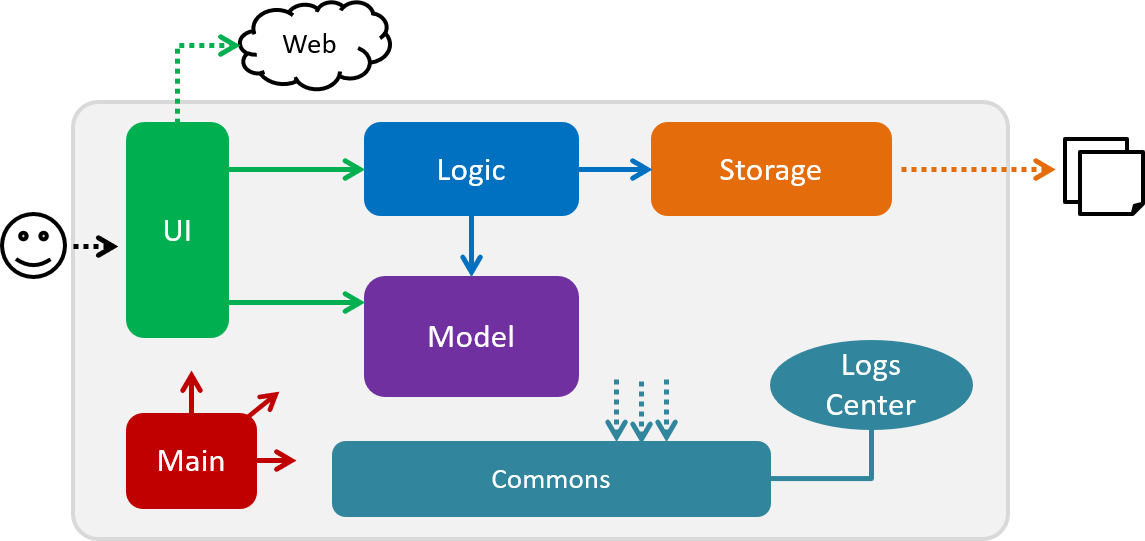
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components.
The following class plays an important role at the architecture level:
-
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
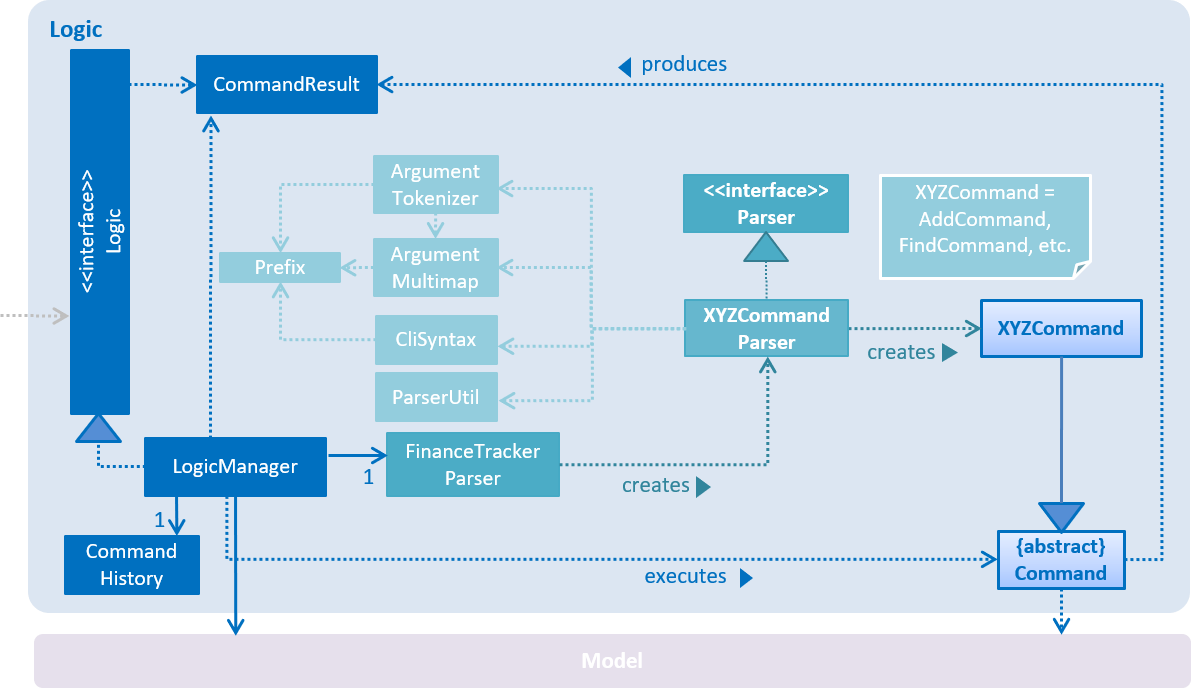
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command deleteexpense 1
.
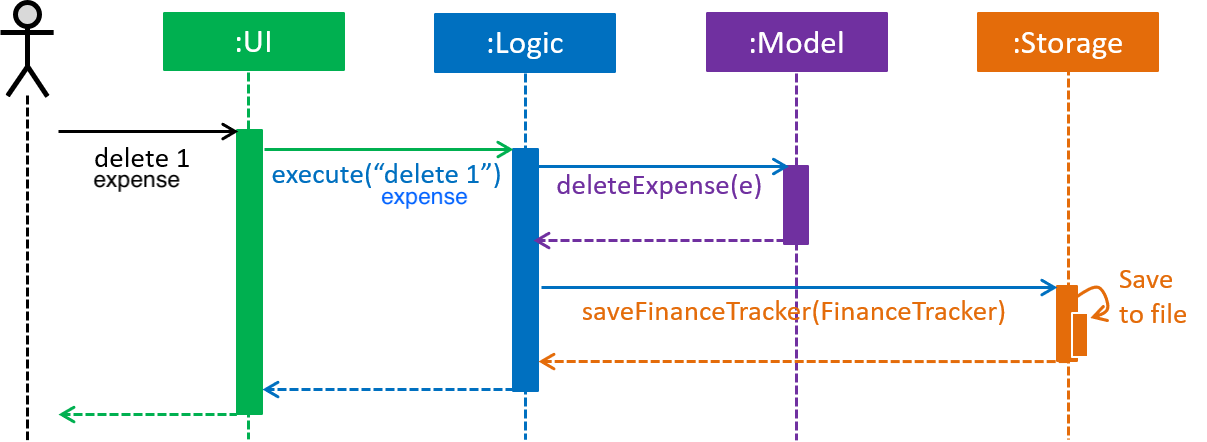
deleteexpense 1
commandThe sections below give more details of each component.
2.2. UI component
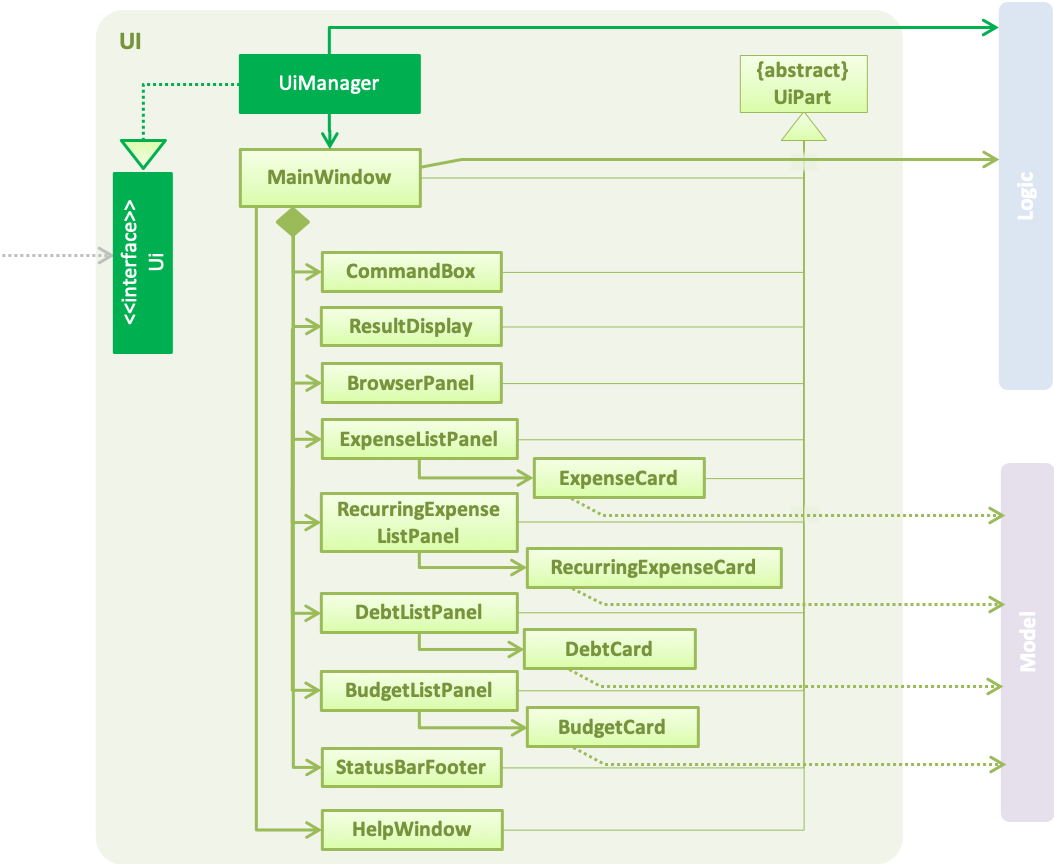
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, ExpenseListPanel
, BudgetListPanel
, DebtListPanel
, RecurringExpenseListPanel
, StatusBarFooter
, BrowserPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class. Each individual ListPanel
consists of their respective Card
.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Listens for changes to
Model
data so that the UI can be updated with the modified data.
2.3. Logic component
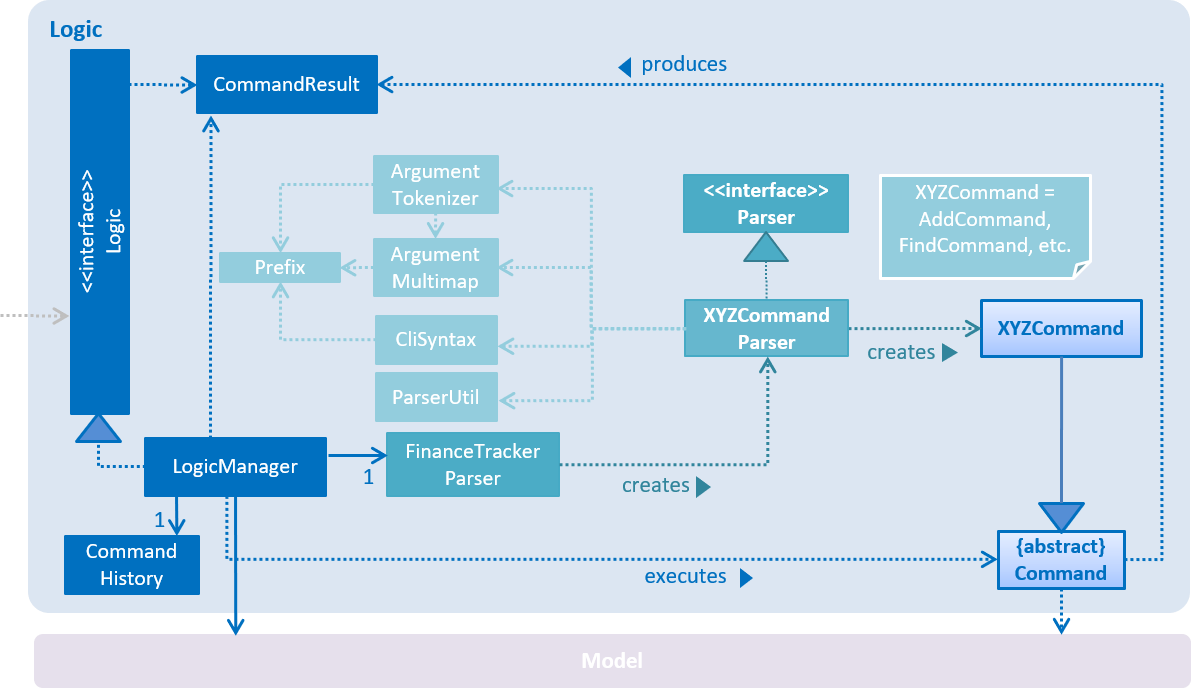
API :
Logic.java
-
Logic
uses theFinanceTrackerParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a expense). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("deleteexpense 1")
API call.
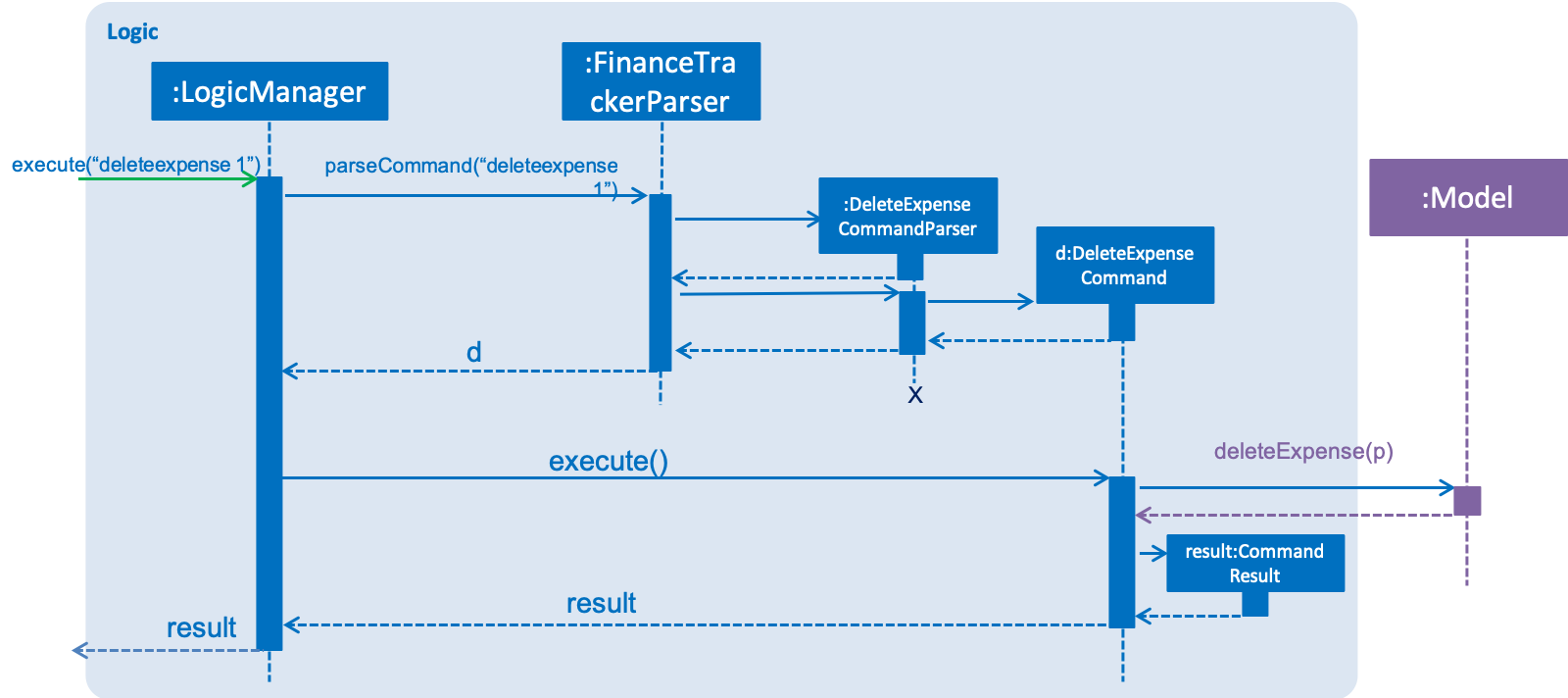
deleteexpense 1
Command2.4. Model component
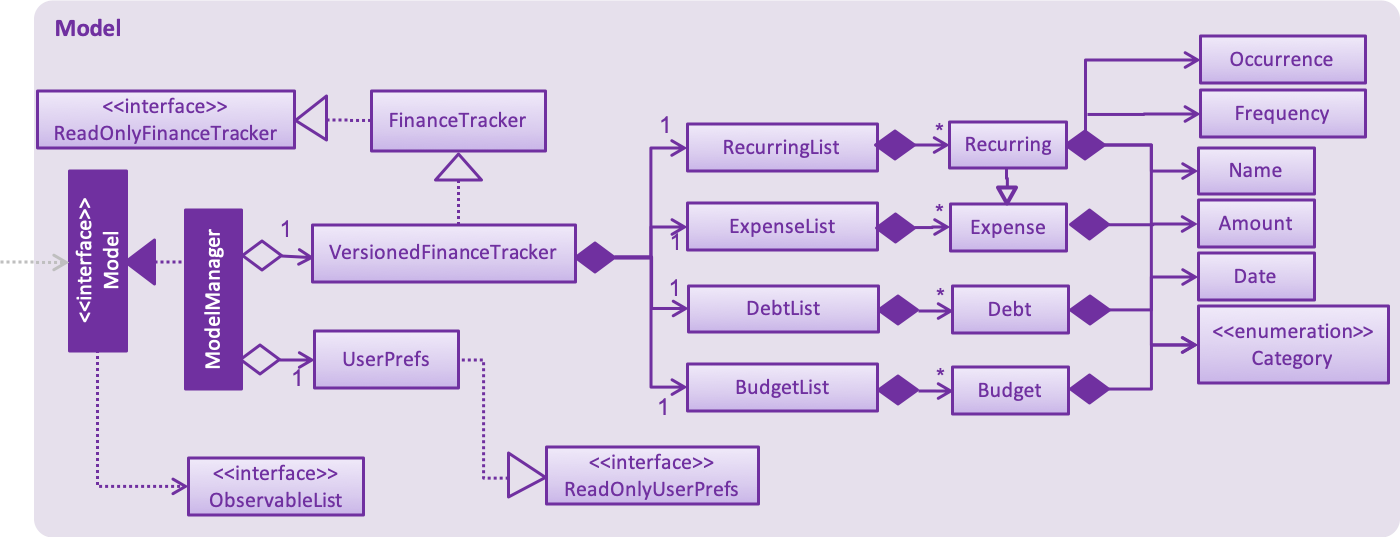
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Finance Tracker data.
-
exposes unmodifiable
ObservableList<Expense>
,ObservableList<Recurring>
,ObservableList<Debt>
,ObservableList<Budget>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
2.5. Storage component
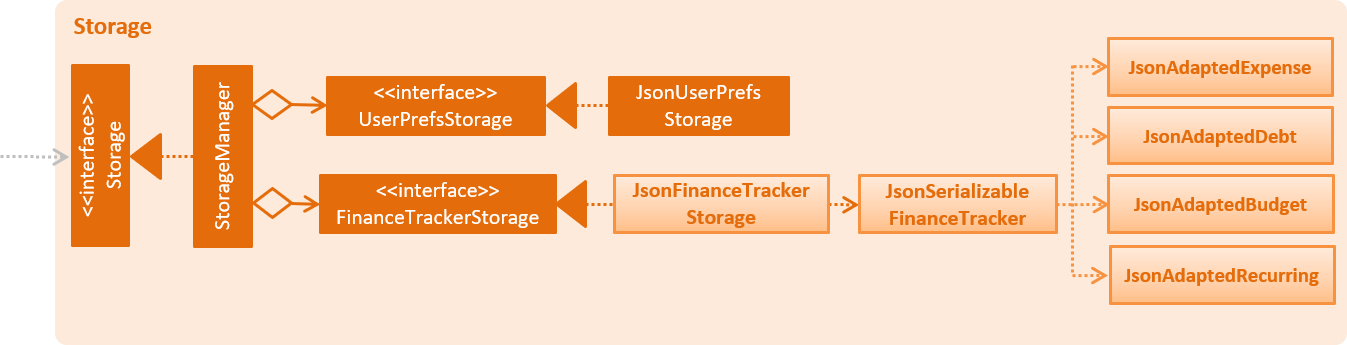
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the Finance Tracker data in json format and read it back.
2.6. Common classes
Classes used by multiple components are in the seedu.address.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. Undo/Redo feature
3.1.1. Current Implementation
The undo/redo mechanism is facilitated by VersionedFinanceTracker
.
It extends FinanceTracker
with an undo/redo history, stored internally as a financeTrackerStateList
and currentStatePointer
.
Additionally, it implements the following operations:
-
VersionedFinanceTracker#commit()
— Saves the current finance tracker state in its history. -
VersionedFinanceTracker#undo()
— Restores the previous finance tracker state from its history. -
VersionedFinanceTracker#redo()
— Restores a previously undone finance tracker state from its history.
These operations are exposed in the Model
interface as Model#commitFinanceTracker()
, Model#undoFinanceTracker()
and Model#redoFinanceTracker()
respectively.
Given below is an example usage scenario and how the undo/redo mechanism behaves at each step.
Step 1. The user launches the application for the first time. The VersionedFinanceTracker
will be initialized with the initial finance tracker state, and the currentStatePointer
pointing to that single finance tracker state.

Step 2. The user executes deleteexpense 5
command to delete the 5th expense in the finance tracker. The deletexpense
command calls Model#commitFinanceTracker()
, causing the modified state of the finance tracker after the deleteexpense 5
command executes to be saved in the financeTrackerStateList
, and the currentStatePointer
is shifted to the newly inserted finance tracker state.
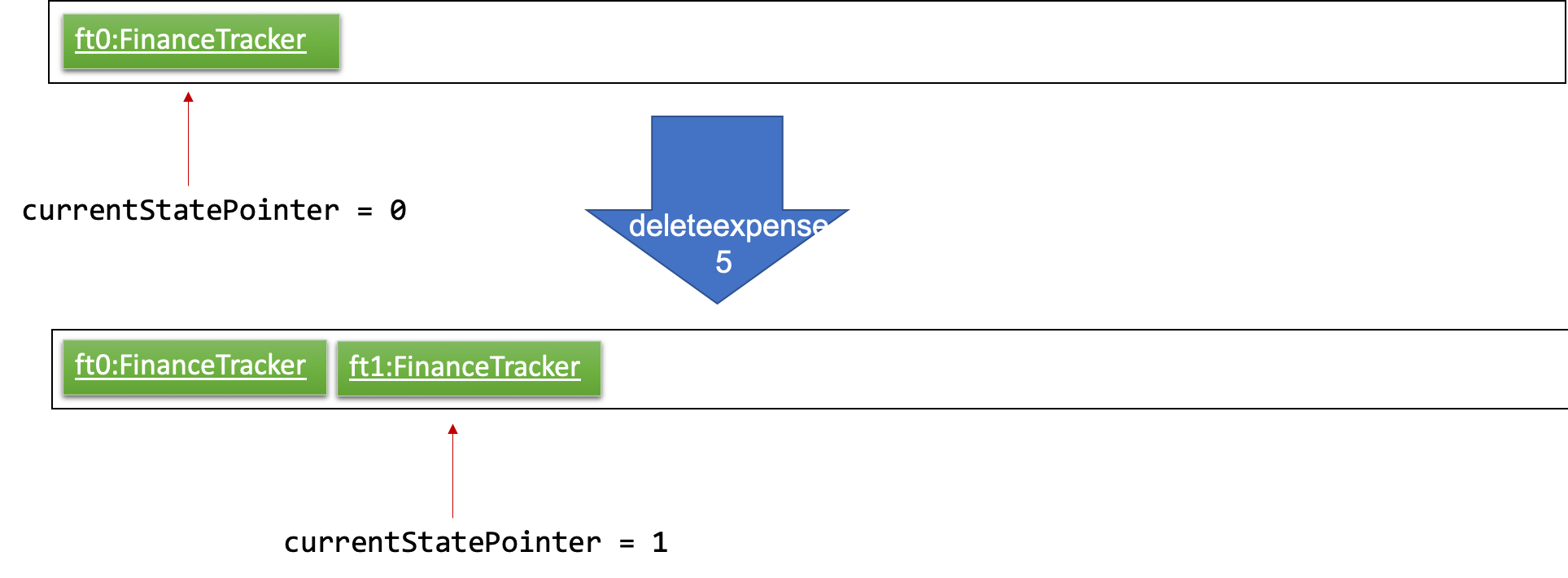
Step 3. The user executes addexpense n/BKT …
to add a new expense. The addexpense
command also calls Model#commitFinanceTracker()
, causing another modified finance tracker state to be saved into the financeTrackerStateList
.
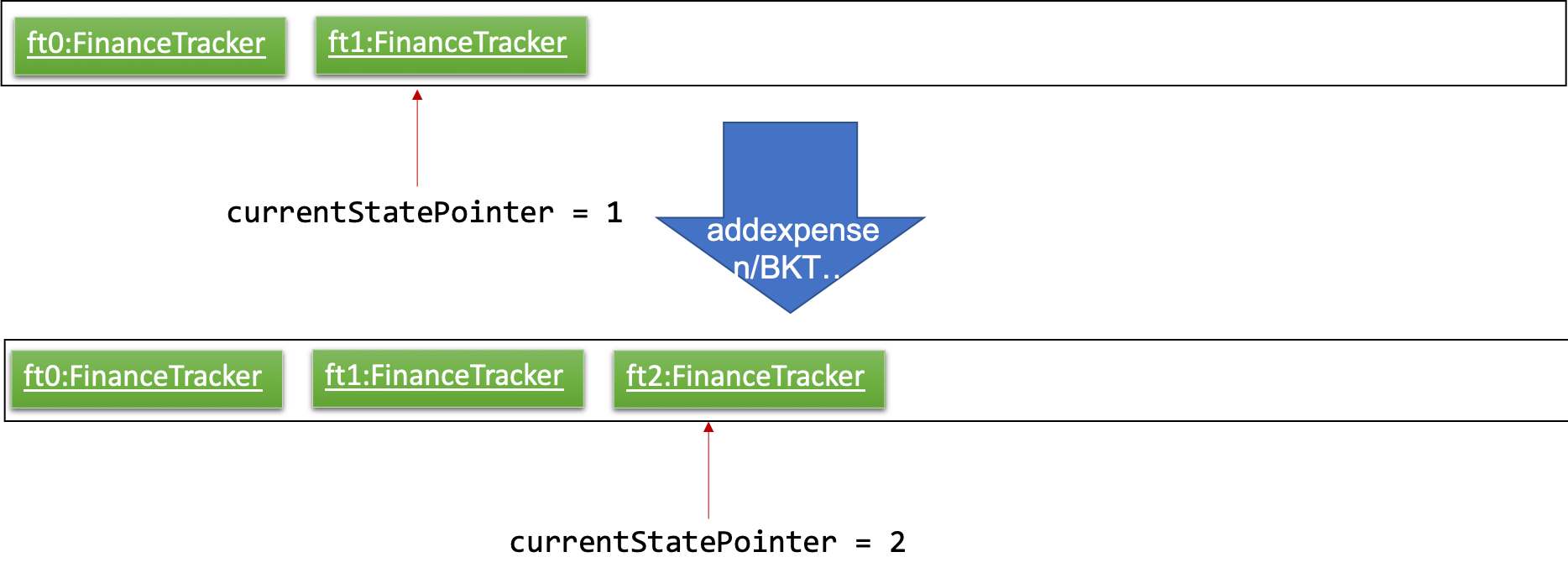
If a command fails its execution, it will not call Model#commitFinanaceTracker() , so the finance tracker state will not be saved into the financeTrackerStateList .
|
Step 4. The user now decides that adding the expense was a mistake, and decides to undo that action by executing the undo
command. The undo
command will call Model#undoFinanceTracker()
, which will shift the currentStatePointer
once to the left, pointing it to the previous finance tracker state, and restores the finance tracker to that state.
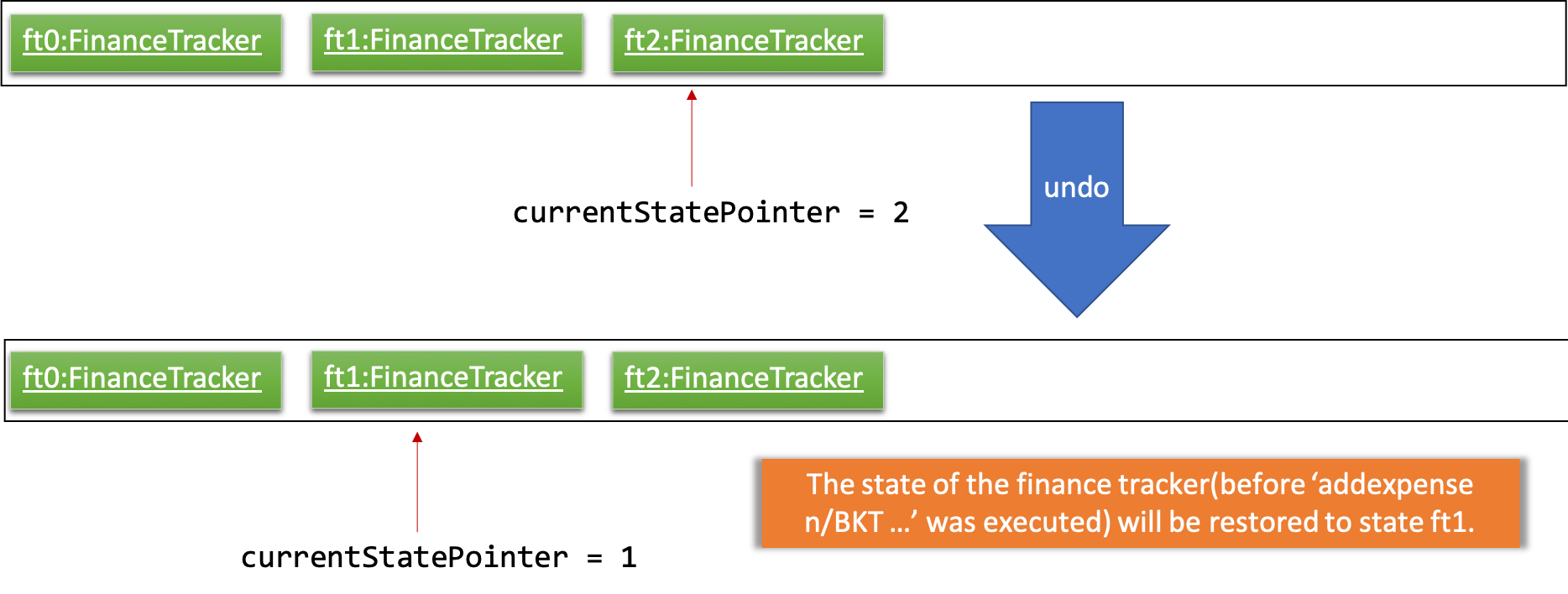
If the currentStatePointer is at index 0, pointing to the initial finance tracker state, then there are no previous finance tracker states to restore. The undo command uses Model#canUndoFinanceTracker() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the undo.
|
The following sequence diagram shows how the undo operation works:
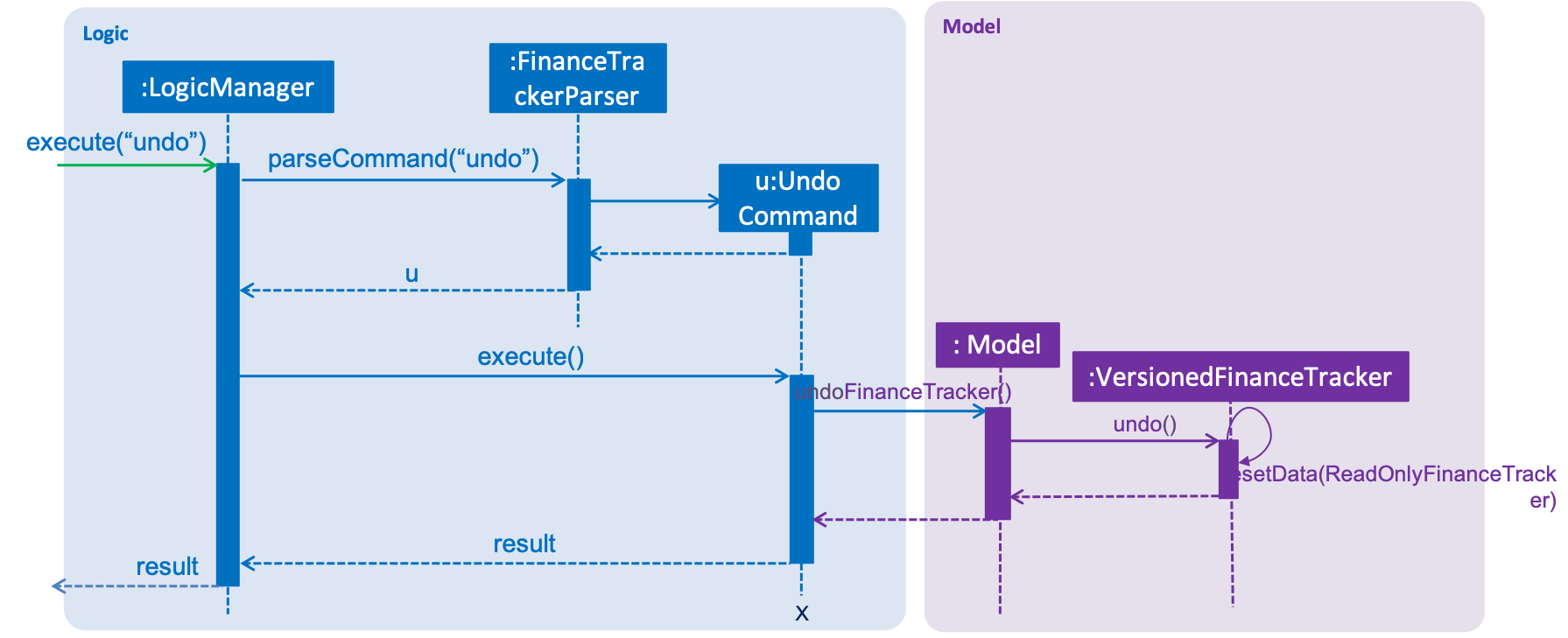
The redo
command does the opposite — it calls Model#redoFinanceTracker()
, which shifts the currentStatePointer
once to the right, pointing to the previously undone state, and restores the finance tracker to that state.
If the currentStatePointer is at index financeTrackerStateList.size() - 1 , pointing to the latest finance tracker state, then there are no undone finance tracker states to restore. The redo command uses Model#canRedoFinanceTracker() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the redo.
|
Step 5. The user then decides to execute the command listexpense v/all
. Commands that do not modify the finance tracker, such as listexpense
, will usually not call Model#commitFinanceTracker()
, Model#undoFInanceTracker()
or Model#redoFinanceTracker()
. Thus, the financeTrackerStateList
remains unchanged.
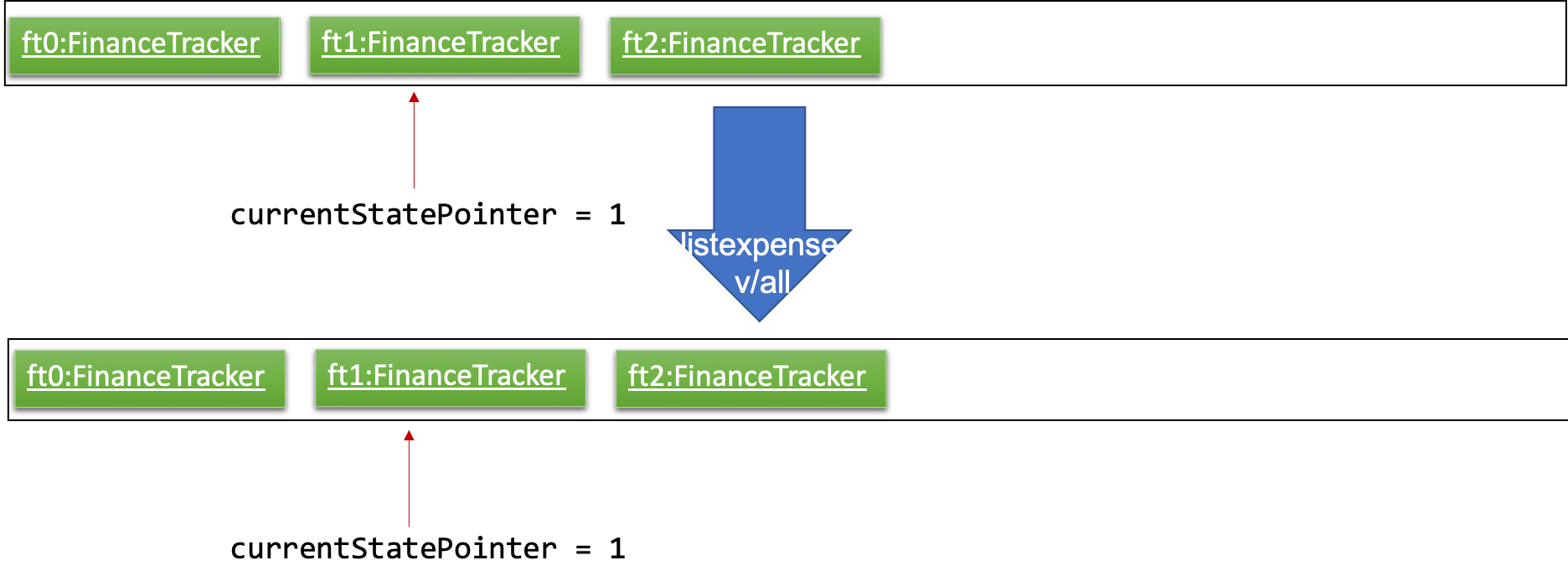
Step 6. The user executes clearexpense
, which calls Model#commitFinanceTracker()
. Since the currentStatePointer
is not pointing at the end of the financeTrackerStateList
, all finance tracker states after the currentStatePointer
will be purged. We designed it this way because it no longer makes sense to redo the addexpense n/BKT …
command. This is the behavior that most modern desktop applications follow.
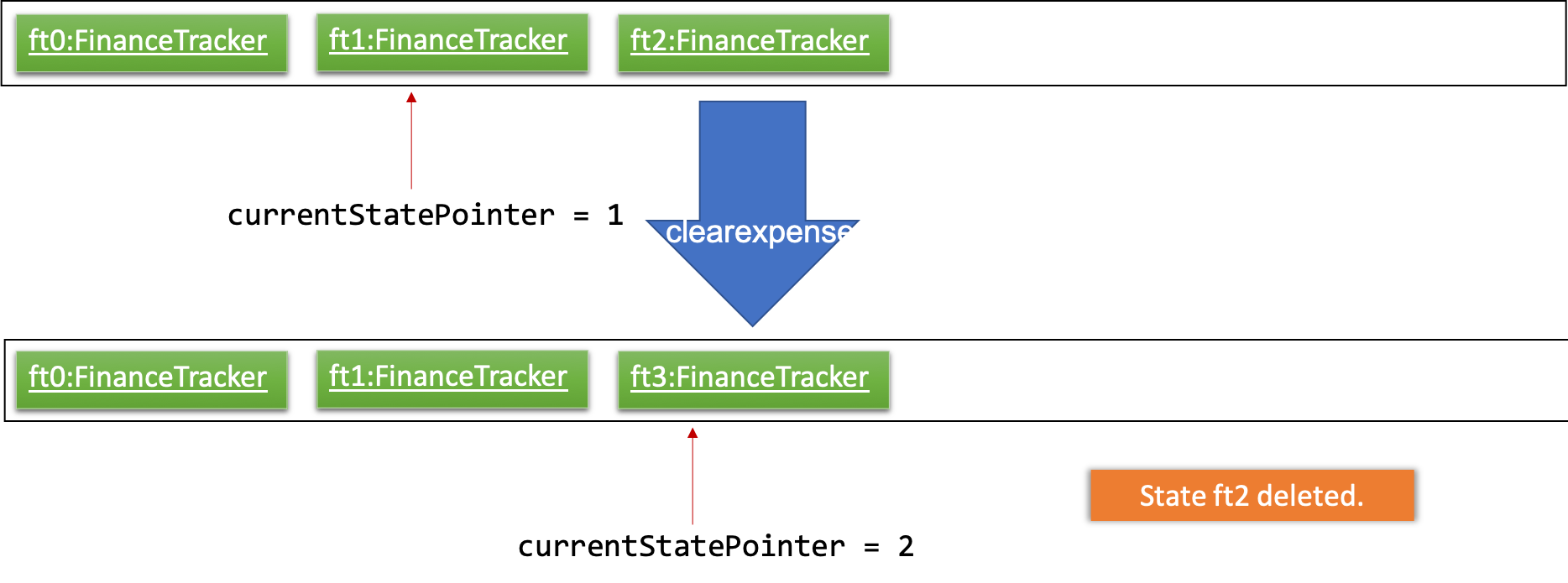
The following activity diagram summarizes what happens when a user executes a new command:
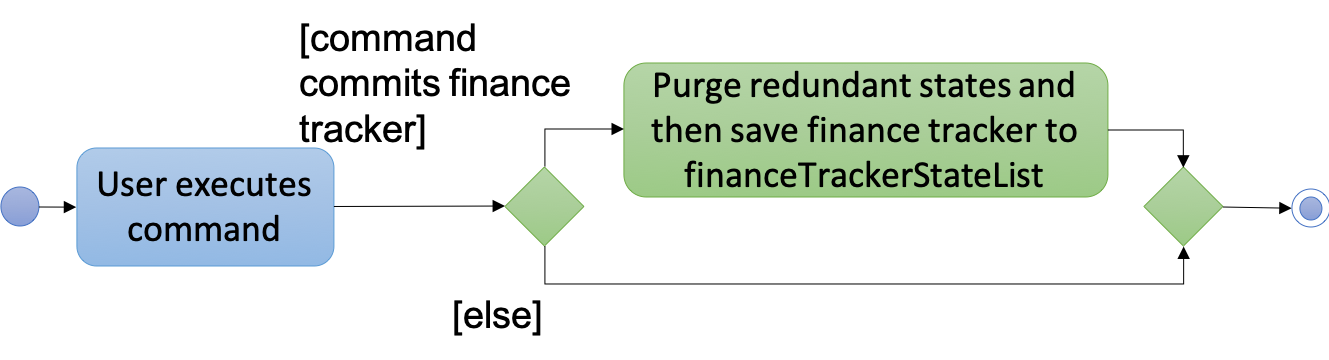
3.1.2. Design Considerations
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire finance tracker.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual command knows how to undo/redo by itself.
-
Pros: Will use less memory (e.g. for
deleteexpense
, just save the expense being deleted). -
Cons: We must ensure that the implementation of each individual command is correct.
-
Aspect: Data structure to support the undo/redo commands
-
Alternative 1 (current choice): Use a list to store the history of finance tracker states.
-
Pros: Easy for new Computer Science student undergraduates to understand, who are likely to be the new incoming developers of our project.
-
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update both
HistoryManager
andVersionedFinanceTracker
.
-
-
Alternative 2: Use
HistoryManager
for undo/redo-
Pros: We do not need to maintain a separate list, and just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Violates Single Responsibility Principle and Separation of Concerns as
HistoryManager
now needs to do two different things.
-
3.2. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 3.3, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.3. Configuration
Certain properties of the application can be controlled (e.g user prefs file location, logging level) through the configuration file (default: config.json
).
3.4. Listing Feature
This feature allows users to filter out specific items based on the view specified. Only expenses that are under those views will be shown on the respective list panels.
Listing feature consists of commands such as listexpense
, listrecurring
and listdebt
.
This implementation is under Logic and Model components.
3.4.1. Current implementation
Below is the UML sequence diagram and a step-by-step explanation of an example usage scenario for listexpense
. The other list commands have similar implementations.
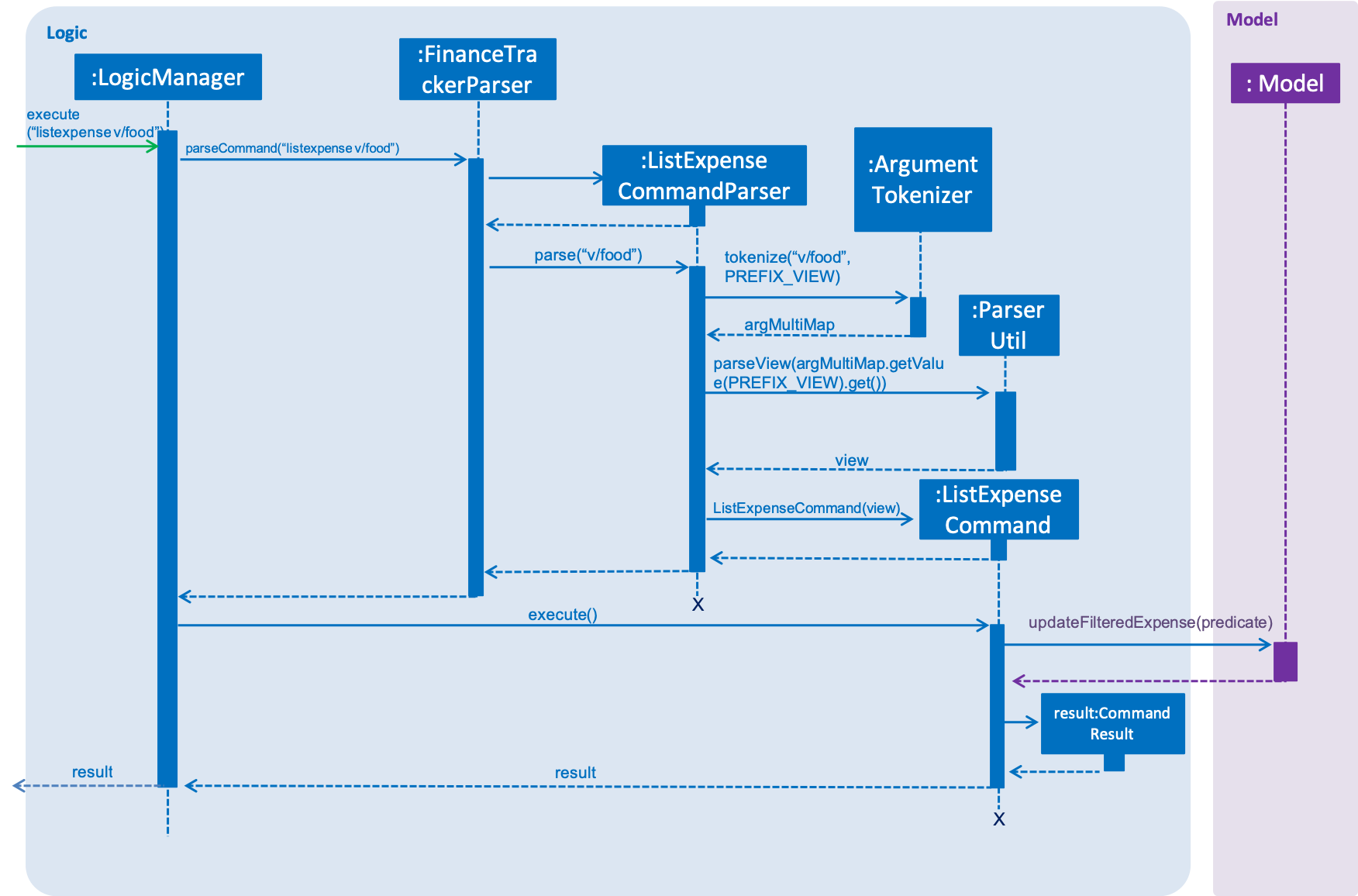
-
User enters command
listexpense v/food
. The command is received byFinanceTrackerParser
, which then creates aListExpenseCommandParser
object. The created object then callsListExpenseCommandParser#parse("v/food")
method. -
ListExpenseCommandParser#parse("v/food")
method calls theArgumentTokenizer#tokenize()
to tokenize the inputv/food
and stores it in anArgumentMultiMap
object. -
ListExpenseCommandParse#parse("v/food")
method then callsParserUtil#parseView(argMultiMap#getValue(PREFIX_VIEW)#get())
and gets aView
object in return. -
After that, a
ListExpenseCommand
object is created with theView
object as parameter and return toLogicManager
. -
LogicManager
then callsListExpenseCommand#execute()
, which callsModel#updateFilteredExpense(predicate)
, where predicate is obtained from calling theListExpenseCommand#getPredicate(view)
. It then updates the filter of the filtered expense list and it now contains the new set of expenses that are filtered by the predicate. -
Finally, the expense list panel will show the new set of expenses. A
CommandResult
is then created and returned toLogicManager
.
3.4.2. Design Consideration
This feature can be implemented in different ways in terms of how the specified expenses are filtered out. The alternative ways of implementation are shown below.
-
Alternative 1 (current choice): Go through all expenses in the
FinanceTracker
and filter out those that are under the specified view.-
Pros: Easy to implement. The original architecture is preserved.
-
Cons: Time-consuming. Large number of expenses in FinanceTracker will take longer time to filter.
-
-
Alternative 2: Store expenses in separate files based on different views. Only check files under the specified view when filtering.
-
Pros: More efficient. Will not go through every single expense.
-
Cons: Will need to alter the original architecture. More storage space will be needed.
-
3.5. Recurring Feature
The Recurring feature consists of addrecurring
, editrecurring
, listrecurring
, deleterecurring
clearrecurring
, and convertrecurring
.
Recurring expenses are expenses that are periodic and have a fixed frequency and a defined number of occurrences. Examples of these recurring expenses are bills or subscription fees.
This feature allows users to add recurring expenses to the finance tracker and be able to constantly keep track of them. This is done by calling Logic#execute
which creates an AddRecurringCommand
. This command then calls Model#addRecurring
, adding the specified recurring into the recurring list.
This feature also simplifies the deletion and editing of these recurring expenses, and they work in a similar manner.
3.5.1. Convert Recurring To Expense Feature
The purpose of this feature is to provide the convenience for users by adding outstanding expenses from all their recurring expenses into the expense list. After adding recurring expenses into the Finance Tracker, the user can call convertrecurring
to make the conversion.
Current Implementation
Below is the UML sequence diagram and a step-by-step explanation of an example usage scenario for convertrecurring
.
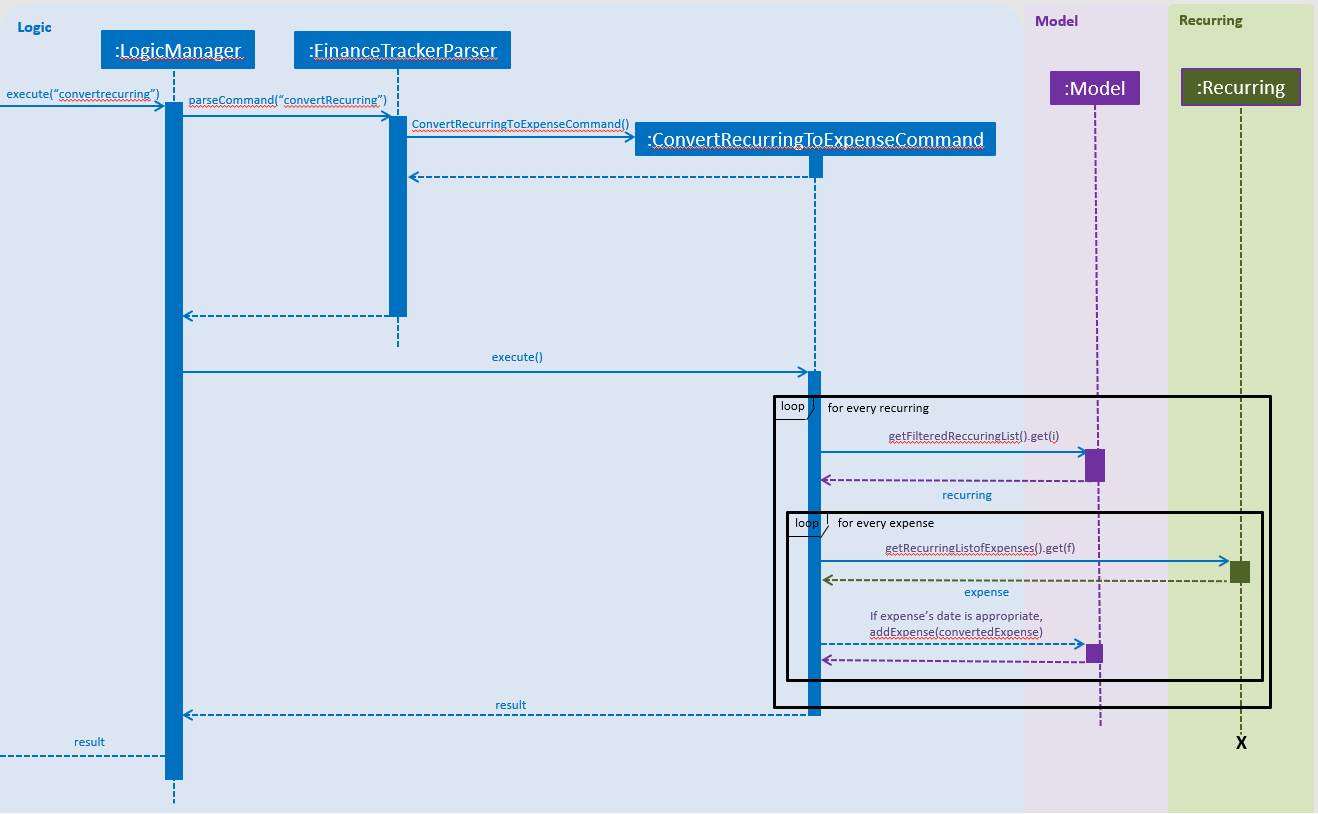
-
User enters the command
convertrecurring
, to add outstanding expenses from all their recurring expenses into the expense list. This command is executed byLogicManager
, which callsFinanceTrackerParser#parseCommand("convertrecurring")
. This creates aConvertRecurringToExpenseCommand
object and returned to theLogicManager
. -
The
LogicManager
callsConvertRecurringToExpenseCommand#execute
, which will call Model#getFilteredRecurringList() to retrieve the list of recurrings in the financetracker. -
The programme then iterates through the recurring list, and for each recurring, checks the recurring’s
lastConvertedDate
, which is the last time the recurring has gone through aconvertrecurring
check. -
If the recurring’s
lastConvertedDate
is before the current date, this recurring will have to be checked again for outstanding expenses that can potentially be added. -
The programme then calls Recurring#getRecurringListofExpenses to retrieve the list of expenses that are supposed to be added by this recurring, and iterates through this expense list.
-
If the expense’s date is before or equals to the current date, and after the
lastConvertedDate
, this recurring expense will have to be converted into an expense and added into the FinanceTracker. This is done by calling Model#addExpense(recurringListOfExpenses.get(j)). -
This is done for every expense that is supposed to be added by this recurring to determine which has to be added into the expense list. After the iteration, the recurring’s
lastConvertedDate
is set to the current date. -
This is done for each recurring in the list.
3.5.2. Advanced Delete and Edit Recurring (coming in v2.0)
This is still a work in progress.
Currently, deleterecurring
does not delete expenses already added by the deleted recurring, and editrecurring
does not edit expenses already added by the edited recurring.
The purpose of this feature is to make deleterecurring
and editrecurring
more advanced such that the expenses already added by the recurring to be deleted or edited will also reflect the new changes.
3.6. Debt Feature
The Debt feature consists of adddebt
, editdebt
, listdebt
, deletedebt
, selectdebt
, cleardebt
and paydebt
.
This feature allows users to add debts to the finance tracker and be able to constantly keep track of them. This is done by calling Logic#execute
which creates an AddDebtCommand
. This command then calls Model#addDebt
, adding the specified debt into the debt list.
Editing, deleting, selecting and clearing of debts work in a similar manner.
3.6.1. Pay Debt Feature
The purpose of this feature is to provide the convenience for users to indicate that they have paid off a particular debt and seamlessly convert that into an expense.
Current Implementation
Below is the UML sequence diagram and a step-by-step explanation of an example usage scenario for paydebt
.
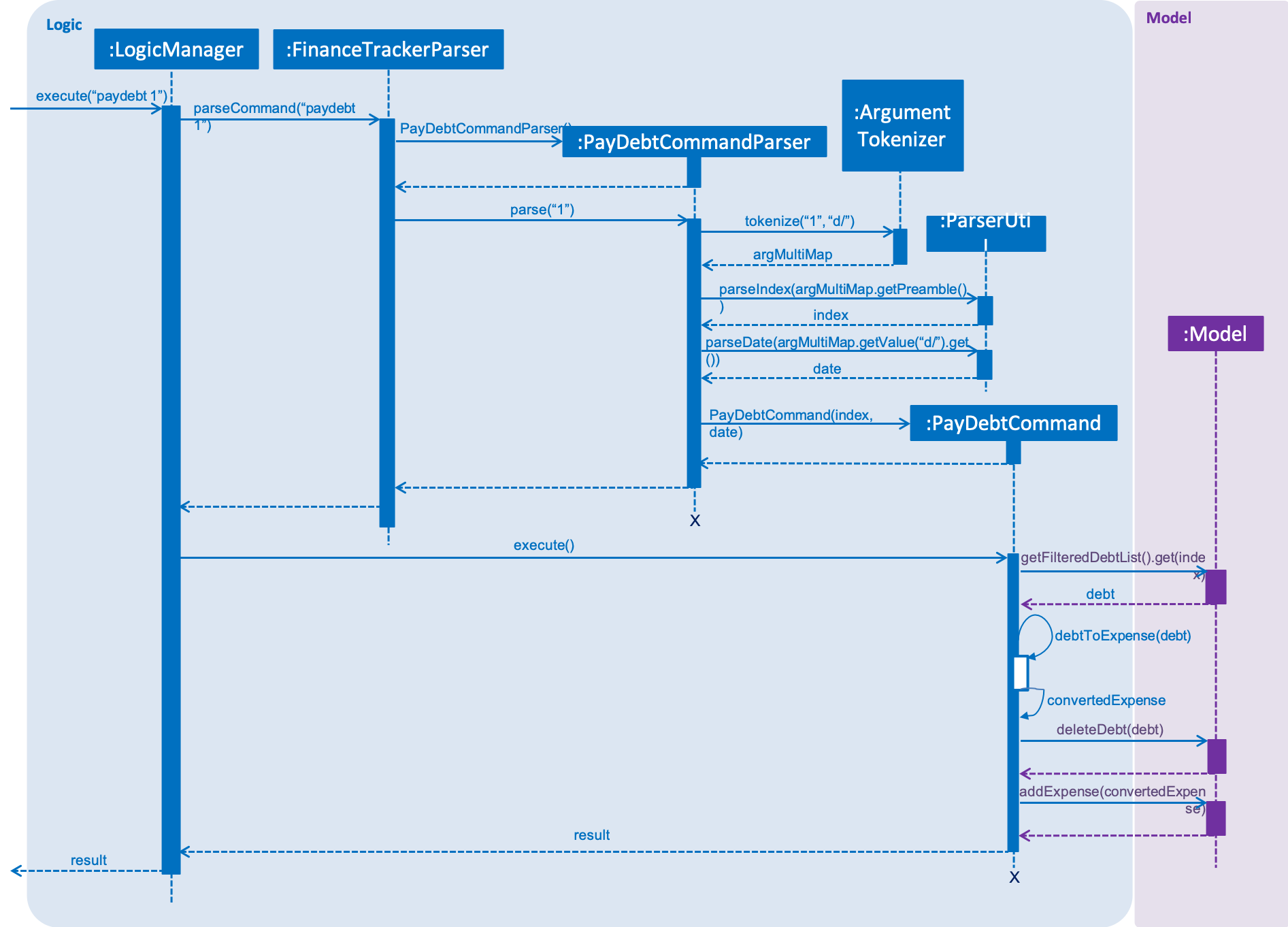
-
User enters the command
paydebt 1
, to convert the first listed debt on the user interface into an expense. This command is executed byLogicManager
, which callsFinanceTrackerParser#parseCommand("paydebt 1")
. This creates a newPayDebtCommandParser
object which will help parse the input by the user. -
The newly created object calls
parse("1")
which in turns callsArgumentTokenizer#tokenize("1", "d/")
to split the arguments into its preamble(the index) and the date(if inputted by the user). This returns anargMultiMap
containing the split input. -
The
PayDebtCommandParser
object then callsParserUtil#parseIndex(argMultiMap.getPreamble())
andParserUtil#parseDate(argMultiMap.getValue("d/").get())
to parse the arguments into the index and date into its correct form respectively. If the user does not input a date, the program will use the local date on the system’s clock. APayDebtCommand
object containing the index and date is then created and returned to theLogicManager
. -
The
LogicManager
callsPayDebtCommand#execute()
, which will callModel#getFilteredDebtList()
to retrieve the list of debts stored in the finance tracker. The methodget(index)
is then called to retrieve thedebt
the user was intending to convert. -
An expense is then created by retrieving out the following relevant information from the
debt
entry:-
Person Owed
-
Amount
-
Category
-
Remarks
-
-
The programme then take this information and creates a new Expense
convertedExpense
with the following fields:-
Name
: Paid Debt toPerson Owed
-
Amount
: Same amount will be used -
Category
: Same category will be used -
Date
: Based on user’s input on when the debt is paid, else the program will use the local date in the system’s clock -
Remarks
: Same remarks will be used
-
-
Model#deleteDebt(debt)
andModel#addExpense(convertedExpense)
are then called to delete the targeteddebt
from the debt list and add theconvertedExpense
into the expense list accordingly. -
The command result is then returned to the
LogicManager
which then returns it to theUI
where the changes are then reflected on the user interface.
3.6.2. Design Consideration
This feature can be implemented in different ways in terms of the architecture of the Model component. The alternative ways of implementation are shown below.
-
Alternative 1 (current choice): Debt and Expense classes do not inherit from a common Interface. Container objects such as expense list and debt list, will be initialized to hold objects of their own individual classes.
-
Pros: Lower level of coupling between Expense and Debt classes.
-
Cons: Slower and less flexibility. Conversion between classes, e.g. from Debt to Expense, requires extracting information from debt, creating a new expense, then deleting the old debt.
-
-
Alternative 2: Debt and Expense classes inherit from a common Interface. Container objects such as expense list and debt list, will be initialized to hold objects of this common Interface.
-
Pros: Greater flexibility. Polymorphism allows for easy conversion between different types of entries, such as from debt to expense, simply by moving them across different lists. This will be useful considering that our Finance Tracker will need significant amounts of conversion between different objects.
-
Cons: Higher level of coupling between Expense and Debt classes.
-
Ultimately, the first option was chosen because a lower level of coupling will allow for easier maintenance, integration and testing. Also, it is not significantly slower thus the Finance Tracker will still be able to complete debt-related commands within 2 seconds, which is one of our non-functional requirements.
3.6.3. Debt Notifications (Coming in v2.0)
This is still a work in progress.
The purpose of this feature is to alert users when the deadline for their debts is approaching. These alerts come in the form of either a pop-up notification or an email alert.
It is up to the user on when they would like to receive this notification. Currently, there are plans to implement the following options:
-
1 month
before debt deadline -
1 week
before debt deadline -
3 days
before debt deadline -
1 day
before debt deadline
Future Implementation
Once the duration
option is set, each time the main application loads, the programme will go through every debt in Model#getFilteredDebtList()
, deduct duration
from each Debt#getDeadline()
to get its notificationDate
and add them to a new list notificationDates
.
Each notificationDate
is then checked against LocalDate#now()
. If notificationDate
is before or equals to LocalDate#now
, they are then added to a new list notificationsToBeAlerted
.
Every item in notificationsToBeAlerted
is then printed on an alert box, reminding the user of the upcoming deadlines for their debts.
3.7. Statistics Feature
This feature allows users to calculate various statistics for the entries in their Financial Tracker. The feature will read the expenses, debts and budgets and generate various metrics to display.
The Statistic feature consists of stats
, statstrend
and statscompare
This implementation is under Logic and Model components.
3.7.1. stats
Current implementation
Below is the UML sequence diagram and a step-by-step explanation of an example usage scenario for stats
.
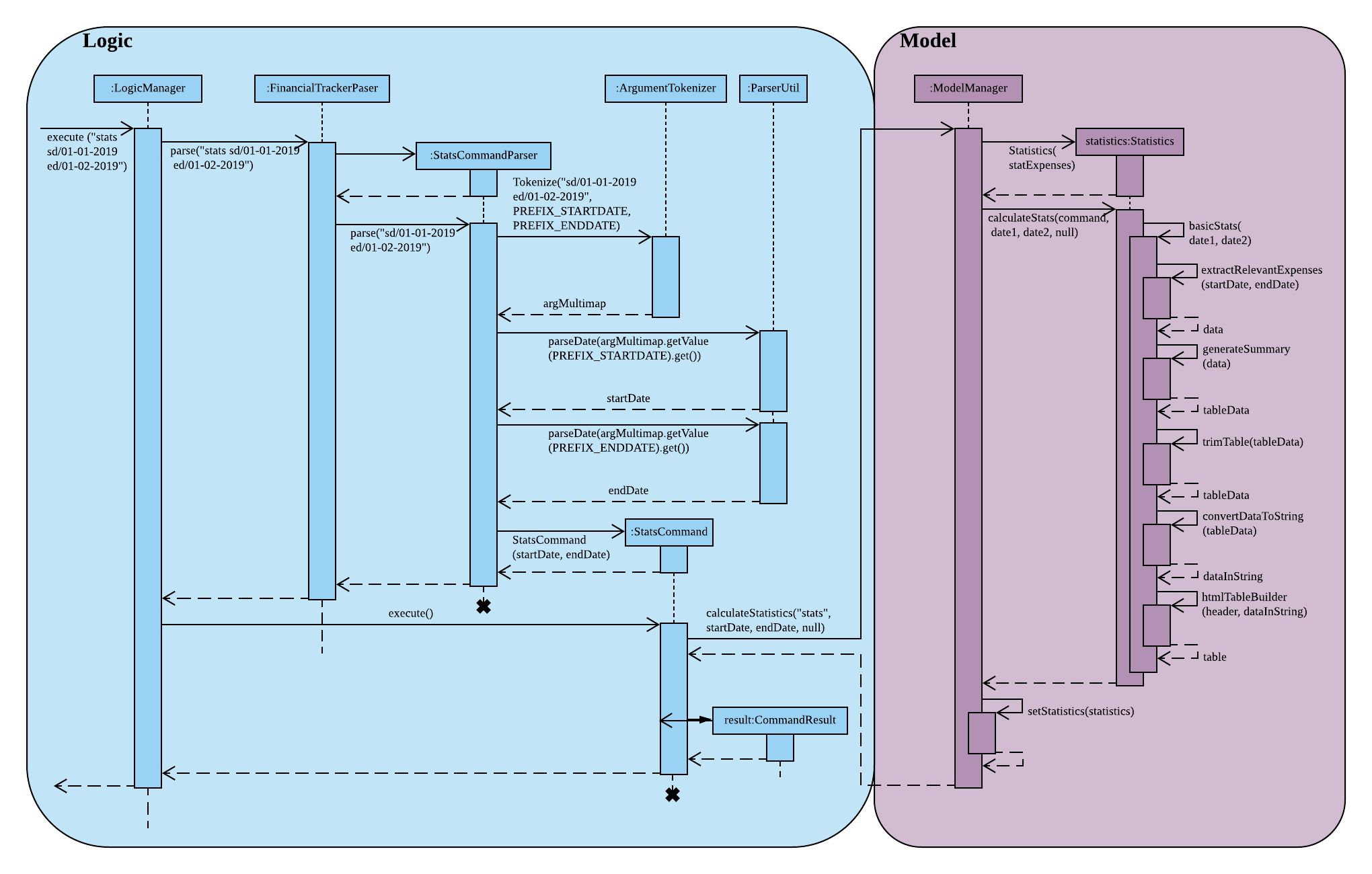
-
User enters command
stats sd/01-01-2019
. The command is received byFinanceTrackerParser
, which then creates aStatsCommandParser
object. The created object then callsStatsCommandParser.parse("stats sd/01-01-2019")
method. AStatsCommand
object is then created withStatsCommand(01-01-2019, 01-02-2019)
and returned to theLogicManager
-
Logic Manager
will call theexecute
method of theStatsCommand
object which calls thecalculateStatistics("stats', 01-01-2019, 01-02-2019, null)
method inModel
. TheModel Manager
will handle this command and duplicate theExpense
list held in the Financial Tracker, creating a snapshot. TheModel Manager
will instantiate aStatistics
object and call thecalculateStatistics("stats', 01-01-2019, 01-02-2019, null)
method. -
The
calculateStatistics
method inStatistics
is a wrapper that will call the appropriate methodbasicStats(01-01-2019, 01-02-2019)
after reading the String"stats"
that was passed in. -
basicStats
calls the methodextractRelevantExpense(01-01-2019, 01-02-2019)
which takes only theExpenses
that lie within the time range and adds them to a newExpense
listdata
. These relevantExpenses
are then processed by thegenerateSummary(data)
method. This method will return a table of relevant statistics. -
The methods
trimTable(table)
,convertDataToString(trimmedtable)
are called to process this table.htmlTableBuilder(header, table)
is called to generate the HTML code required to show the Statistics in the BrowserPanel. -
Model Manager
callssetStatistics(statistics)
which sets the observableStatistics
inModelManager
. -
The listener in
BrowserPanel
will detect the change and display the HTML page showing the statistics
Future Implementation
Users will be given the option to choose a Visual Representation method. This will determine the output format of the statistics. Currently, statistics can only be displayed in table format. However, in the future a feature will be implemented to show the statistics in graphical or pictorial formats. These could include pie charts, bar charts, line graphs, etc.
3.8. Budget Feature
This feature allows users to set budgets for different categories so they can limit the amount spent for each category.
It stores all budgets added by the user and keeps track of the expenses added within the user-specified time frame.
This feature includes addbudget
, editbudget
, deletebudget
, selectbudget
and clearbudget
.
The implementation of the budget feature is under the Logic and Model components.
3.8.1. Current implementation
Adding a budget:
User enters the command word addbudget
, followed by the category for which they want to set the budget for, the amount they want to limit themselves to for that category and specifies the start and end date for that budget.
Editing an existing budget:
User enters the command word editbudget
, followed by the category for which they want to edit the budget of and the relevant parameters of which they wish to edit the values of.
Deleting an existing budget:
User enters the command word deletebudget
, followed by the category for which they wish to delete the budget for.
Selecting an existing budget:
User enters the command word selectbudget
, followed by the category for which they wish to select the budget for viewing.
For all four actions, the user command gets parsed by the respective parsers: AddBudgetCommandParser
, EditBudgetCommandParser
, DeleteBudgetCommandParser
and SelectBudgetCommandParser
, which handle the execution of the commands.
Below is the UML sequence diagram of a scenario for the addbudget
command. Editing, deleting and selecting budgets work similarly to adding budgets.
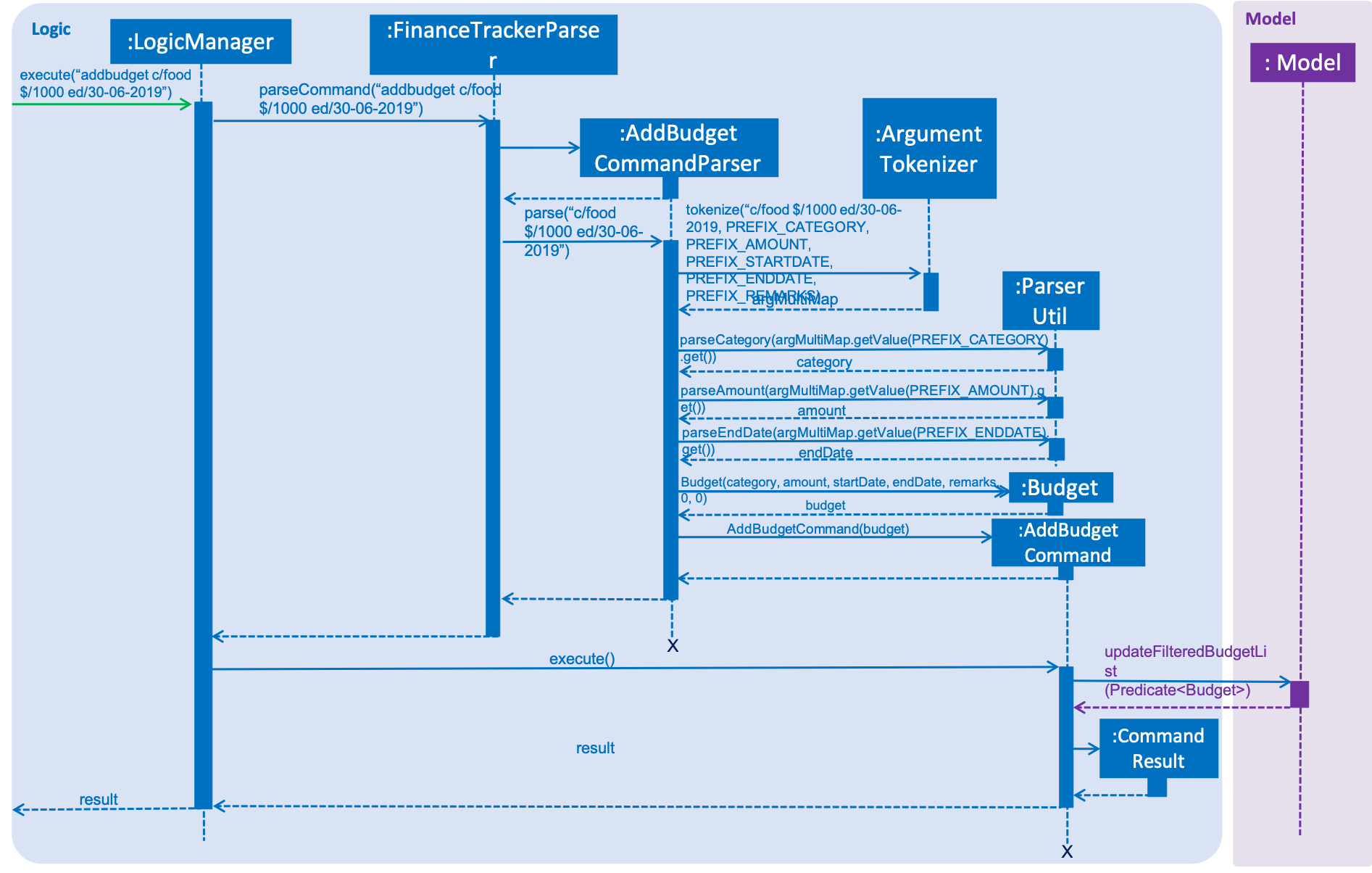
-
User enters the command
addbudget c/food $/1000 ed/30-06-2019
, to add a new budget for theFOOD
category. This command is executed byLogicManager
, which callsFinanceTrackerParser#parseCommand("addbudget c/food $/1000 ed/30-06-2019")
. This creates a newAddBudgetCommandParser
object which will help parse the input by the user. -
The newly created object calls
parse("c/food $/1000 ed/30-06-2019")
which in turn callsArgumentTokenizer#tokenize("c/food $/1000 ed/30-06-2019", "/c", "$/", "sd/", "ed/", "r/")
to split the arguments into the different parameters of a budget. This returns anargMultiMap
containing the split input. -
The
AddBudgetCommandParser
object then callsParserUtil#parseCategory(argMultiMap.getValue("c/").get())
ParserUtil#parseAmount(argMultiMap.getValue("$/").get())
andParserUtil#parseDate(argMultiMap.getValue("ed/").get())
to parse the arguments provided by the user into the correct formats of the relevant parameters. As the user does not specify a start date in this case, the programme will use the local date on the system’s clock. AnAddBudgetCommand
object containing the parameter values provided and today’s date as the start date is then created and returned to theLogicManager
. -
The
LogicManager
callsAddBudgetCommand#execute()
, which will callModel#updateFilteredBudgetList(Predicate<Budget>)
to update the list of budgets stored in the finance tracker. -
Model#addBudget(budget)
is then called to add thebudget
into the budget list. -
The command result is then returned to the
LogicManager
which then returns it to theUI
where the changes are then reflected on the user interface.
Updating of budgets:
-
When a new budget is added, the model checks the expense list for any expenses of that category which fall within the time frame of the added budget and sets the
totalSpent
andpercentage
variables of the Budget object accordingly. -
When an existing budget is edited, the model updates the
percentage
variable of the Budget object. -
For every expense the user enters, the model will check if there exists a budget for the category of the added expense and if the date of the expense is within the time frame of the budget. If the added expense is during the time of the budget, the totalSpent variable in the Budget object with that specific category will be updated.
-
The total amount spent for each budget category, along with the percentage of the total budgeted amount, will be displayed to the user so that the user can know how much has been spent so far and can better plan their future expenses so as not to exceed their budget.
3.8.2. Future Enhancements
The Budget class has a boolean method overlaps(Budget other)
which returns true if two budgets of the same category have overlapping time frames.
This method was included with the idea of allowing the user to add multiple budgets for the same category but for different time frames.
Another future enhancement would be to notify the user when a certain budget is about to exceed. For example, if the percentage spent within the time frame reaches say 90% of the budget amount, the user will receive an e-mail or notification of some sort to alert the user. Another notification can also be sent when the budget is actually exceeded.
Yet another possible enhancement would be to allow the user to set a recurring budget.
For example, if a user wants to limit their daily spending to $20, maybe an addrecurringbudget c/food $/20 f/d
command, similar to the recurring expense command, could be implemented so that the user does not have to add a budget everyday.
4. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
4.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
4.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
4.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
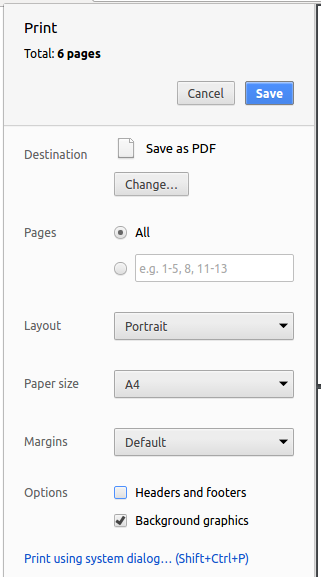
4.4. Site-wide Documentation Settings
The build.gradle
file specifies some project-specific asciidoc attributes which affects how all documentation files within this project are rendered.
Attributes left unset in the build.gradle file will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
The name of the website. If set, the name will be displayed near the top of the page. |
not set |
|
URL to the site’s repository on GitHub. Setting this will add a "View on GitHub" link in the navigation bar. |
not set |
|
Define this attribute if the project is an official SE-EDU project. This will render the SE-EDU navigation bar at the top of the page, and add some SE-EDU-specific navigation items. |
not set |
4.5. Per-file Documentation Settings
Each .adoc
file may also specify some file-specific asciidoc attributes which affects how the file is rendered.
Asciidoctor’s built-in attributes may be specified and used as well.
Attributes left unset in .adoc files will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
Site section that the document belongs to.
This will cause the associated item in the navigation bar to be highlighted.
One of: * Official SE-EDU projects only |
not set |
|
Set this attribute to remove the site navigation bar. |
not set |
4.6. Site Template
The files in docs/stylesheets
are the CSS stylesheets of the site.
You can modify them to change some properties of the site’s design.
The files in docs/templates
controls the rendering of .adoc
files into HTML5.
These template files are written in a mixture of Ruby and Slim.
Modifying the template files in |
5. Testing
5.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
5.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.address.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.address.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.address.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.address.logic.LogicManagerTest
-
5.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
HelpWindow.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
6. Dev Ops
6.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
6.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
6.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
6.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
6.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
6.6. Managing Dependencies
A project often depends on third-party libraries. For example, Finance Tracker depends on the Jackson library for JSON parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives:
-
Include those libraries in the repo (this bloats the repo size)
-
Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Suggested Programming Tasks to Get Started
Suggested path for new programmers:
-
First, add small local-impact (i.e. the impact of the change does not go beyond the component) enhancements to one component at a time.
-
Next, add a feature that touches multiple components to learn how to implement an end-to-end feature across all components.
Appendix B: Product Scope
Target user profile:
-
has a need to manage a significant number of expenses, debts and bills/subscriptions
-
wants to keep track of how much is being spent
-
prefer desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: manage finances faster than a typical mouse/GUI driven app
Appendix C: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a/an … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use the App |
|
user |
view a list of my expenses in certain time periods |
have a better overview of my expenses |
|
user |
add expenses into the finance tracker |
record all my expenses |
|
user |
see the total amount of money I have |
see how much money I have left overall and better plan my finances |
|
user |
make changes to expenses I previously added |
correct any mistakes |
|
organised user |
categorise my expenses |
know my spending for each category |
|
user |
delete an expense |
remove entries that I no longer need to keep track of |
|
thrifty user |
set a budget |
manage my expenses and see how much more I can spend for the remaining part of the day/week/month/year |
|
user |
split my budget into different categories |
enhance my finance planning further and not spend excessively on a single category, e.g. food |
|
user |
edit my budget |
change the amount of budget available or the duration |
|
user |
cancel my budget |
|
|
user |
view a list of my debts and see when they are due |
remind myself to pay the people I owe by their due dates |
|
forgetful user |
add my debts |
can see who I owe money to |
|
user |
edit my debts |
change the amount I owe if any changes occur |
|
user |
delete my debts |
|
|
user |
clear my paid debts |
know I have settled them |
|
user |
add my recurring expenses |
keep track of my subscriptions and bills |
|
user |
edit my recurring expenses |
change the details of my bills if any changes occur |
|
user |
delete my recurring expenses |
|
|
analytical user |
see a breakdown of my expenses |
know what I spend the most on |
|
visual user |
have charts and graphs to represent my expenditure |
have a better picture on the different categories of my expenses |
|
efficient user |
be able to view my command history |
easily input a repeated command when needed |
|
user |
undo and redo my actions |
|
|
user |
clear all entries |
start with an empty finance tracker again when needed |
|
non-tech-savvy user |
type simple commands step by step |
use the programme more easily without having to keep referring to the help menu |
|
user |
receive reminders regarding my expenditure |
know if my spending is about to exceed my budget or when my debts are about to be due |
Appendix D: Use Cases
(For all use cases below, the System is the Personal Finance Tracker (PFT)
and the Actor is the user
, unless specified otherwise)
Use case 1: List expenses
MSS
-
User chooses to list all expenses and specifies if user wants to view by specific duration or category
-
PFT displays all expenses according to specified duration or category
Use case ends.
Extensions:
-
1a. PFT detects invalid value for view
-
1a1. PFT informs user that input is invalid
-
Use case ends.
-
Use case 2: Add expense
MSS
-
User chooses to add an expense
-
PFT prompts user for name of expense
-
User enters name
-
PFT prompts user for amount for expense
-
User enters amount
-
PFT prompts user for date of expense
-
User enters date
-
PFT prompts user for remark of expense
-
User enters remark
-
PFT creates expense and displays confirmation
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 10.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9)a. PFT detects wrong format or incorrect data
-
3(5,7,9)a1. PFT requests for correct format
-
3(5,7,9)a2. User enters correct data
-
Use case resumes from step 4(6,8,10)
-
Use case 3: Edit expense
MSS
-
User chooses to edit an existing expense by specifying its index
-
PFT prompts user for new name of expense
-
User enters new name
-
PFT prompts user for new amount of expense
-
User enters new amount
-
PFT prompts user for new category of expense
-
User enters new category
-
PFT prompts user for new date of expense
-
User enters new date
-
PFT prompts user for new remark for expense
-
User enters new remark
-
PFT updates the existing values to the values entered by user
Use case ends
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 12.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9,11)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11)a1. PFT requests for correct format
-
3(5,7,9,11)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12)
-
Use case 4: Delete expense
MSS
-
User chooses to delete an existing expense and specifies its index
-
PFT deletes the expense at the specified index
Use case ends.
Extensions:
-
1a. PFT detects that index is invalid.
-
1a1. PFT informs user that index is invalid.
-
Use case ends.
-
Use case 5: Add budget
MSS
-
User chooses to add a budget
-
PFT prompts user for category of budget
-
User enters category
-
PFT prompts user for amount for budget
-
User enters amount
-
PFT prompts user for start date of budget
-
User enters start date
-
PFT prompts user for end date of budget
-
User enters end date
-
PFT creates budget for specified time frame
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 10.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user that command is invalid
-
Use case ends.
-
-
3(5,7,9)a. PFT detects wrong format or incorrect data
-
3(5,7,9)a1. PFT requests for correct format
-
3(5,7,9)a2. User enters correct data
-
Use case resumes from step 4(6,8,10).
-
Use case 6: Edit budget
MSS
-
User chooses to edit an existing budget
-
PFT prompts user for category to edit budget for
-
User enters category
-
PFT prompts user for new amount for budget
-
User enters new amount
-
PFT prompts user for new start date of budget
-
User enters new start date
-
PFT prompts user for new end date of budget
-
User enters new end date
-
PFT updates the existing values to the values entered by user
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 10.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9)a. PFT detects wrong format or incorrect data
-
3(5,7,9)a1. PFT requests for correct format
-
3(5,7,9)a2. User enters correct data
-
Use case resumes from step 4(6,8,10).
-
Use case 7: Delete budget
MSS
-
User chooses to delete an existing budget and specifies its category
-
PFT deletes the budget for the specified category
Use case ends.
Extensions:
-
1a. PFT detects invalid category
-
1a1. PFT informs user that category is invalid
-
Use case ends.
-
Use case 8: List debt
MSS
-
User chooses to list debts
-
PFT lists debts
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 2
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
Use case 9: Add debt
MSS
-
User chooses to add a debt
-
PFT prompts user for expense owed for debt
-
User enters name
-
PFT prompts user for amount owed of debt
-
User enters amount
-
PFT prompts user for category of debt
-
User enters category
-
PFT prompts user for deadline of debt
-
User enters deadline
-
PFT prompts user for remark of debt
-
User enters remark
-
PFT creates budget for specified time frame
Use case ends
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 12.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9,11)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11)a1. PFT requests for correct format
-
3(5,7,9,11)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12).
-
Use case 10: Edit budget
MSS
-
User chooses to edit an existing debt
-
PFT prompts user for new expense owed for debt
-
User enters new name
-
PFT prompts user for new amount for debt
-
User enters new amount
-
PFT prompts user for new category for debt
-
User enters new category
-
PFT prompts user for new end deadline for debt
-
User enters new deadline
-
PFT prompts user for new remark of debt
-
User enters new remark
-
PFT updates the existing values to the values entered by user
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 12.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9,11)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11)a1. PFT requests for correct format
-
3(5,7,9,11)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12).
-
Use case 11: Delete debt
MSS
-
User chooses to delete an existing debt and specifies its index
-
PFT deletes the debt at the specified index
Use case ends.
Extensions:
-
1a. PFT detects invalid index
-
1a1. PFT informs user that index is invalid
-
Use case ends.
-
Use case 12: Pay debt
MSS
-
User chooses to pay off an existing debt and specifies its index
-
PFT converts the debt into an expense entry
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 2.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
Use case 13: Add recurring expense
MSS
-
User chooses to add recurring expense
-
PFT prompts user for name of recurring expense
-
User enters name
-
PFT prompts user for amount of recurring expense
-
User enters amount
-
PFT prompts user for category of recurring expense
-
User enters category
-
PFT prompts user for frequency of recurring expense
-
User enters frequency
-
PFT prompts user for number of occurrences of recurring expense
-
User enters number of occurrences
-
PFT prompts user for start date of recurring expence
-
User enters start date
-
PFT prompts user for remark of recurring expense
-
User enters remark
-
PFT creates recurring expense and displays confirmation
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 16.
-
-
1b. User enters complex command with missing parameters
-
Use case ends.
-
-
3(5,7,9,11,13,15)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11,13,15)a1. PFT requests for correct format
-
3(5,7,9,11,13,15)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12,14,16).
-
Use case 14: Edit recurring expense
MSS
-
User chooses to edit existing recurring expense
-
PFT prompts user for new name of recurring expense
-
User enters new name
-
PFT prompts user for new amount of recurring expense
-
User enters new amount
-
PFT prompts user for new category of recurring expense
-
User enters new category
-
PFT prompts user for new frequency of recurring expense
-
User enters new frequency
-
PFT prompts user for new number of occurrences of recurring expense
-
User enters new number of occurrences
-
PFT prompts user for new start date of recurring expense
-
User enters new start date
-
PFT prompts user for new remark of recurring expense
-
User enters new remark
-
PFT updates parameters with new values
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 16.
-
-
1b. User enters complex command with missing parameters
-
Use case ends.
-
-
3(5,7,9,11,13,15)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11,13,15)a1. PFT requests for correct format
-
3(5,7,9,11,13,15)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12,14,16).
-
Use case 15: Delete recurring expense
MSS
-
User chooses to delete existing recurring expense and specifies both its index and whether to delete all old expenses already added by this recurring expense
-
PFT deletes the recurring expense at the specified index
Use case ends.
Extensions:
-
1a. PFT detects invalid index.
-
1a1. PFT informs user that index is invalid
-
Use case ends.
-
-
1b. User enters command with missing or invalid parameters
-
1b1. PFT informs user of invalid input.
-
Use case ends.
-
Use case 16: View statistics summary
MSS
-
User chooses to view statistics
-
PFT prompts user for start date
-
User enters start date
-
PFT prompts user for end date
-
User enters end date
-
PFT prompts user for visual representation method
-
User enters visual representation method
-
PFT displays the statistics requested
Use case ends
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 8
-
-
1b. User enters complex command with missing parameters
-
1b1. Use case ends
-
-
3(5)a. PFT detects wrong format or incorrect data
-
3(5)a1. PFT requests for correct format
-
3(5)a2. User enters correct data
-
Use case resumes from step 4(6)
-
Use case 17: View statistics trend
MSS
-
User chooses to view statistics trend
-
PFT prompts user for start date
-
User enters start date
-
PFT prompts user for end date
-
User enters end date
-
PFT prompts user for period
-
User enters period
-
PFT displays the statistics requested
Use case ends
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 8.
-
-
1b. User enters complex command with missing parameters
-
Use case ends.
-
-
3(5,7)a. PFT detects wrong format or incorrect data
-
3(5,7)a1. PFT requests for correct format
-
3(5,7)a2. User enters correct data
-
Use case resumes from step 4(6,8).
-
Use case 18: Compare 2 statistic summaries
MSS
-
User chooses to compare statistics
-
PFT prompts user for date 1
-
User enters date
-
PFT prompts user for date 2
-
User enters date
-
PFT prompts user for period
-
User enters period
-
PFT displays the statistics requested
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 8.
-
-
1b. User enters complex command with missing parameters
-
Use case ends.
-
-
3(5,7)a. PFT detects wrong format or incorrect data
-
3(5,7)a1. PFT requests for correct format
-
3(5,7)a2. User enters correct data
-
Use case resumes from step 4(6,8)
-
Use case 19: View command history
MSS
-
User chooses to view command history
-
PFT lists all valid commands entered in reverse chronological order
Use case ends.
Use case 20: Auto-fill previous command
MSS
-
User keys in “Up” arrow
-
PFT auto-fills previous command stored in history in the command line
Steps 1-2 can be repeated as many times as required until the command wanted is reached
-
User presses enter
-
PFT executes autofilled command
Use case ends.
Extensions:
-
2a. User realises user has accidentally pressed "Up" arrow too many times
-
2a1. User presses "Down" arrow
-
2a2. PFT auto-fills next command stored in history in the command line
-
Steps 2a1-2a2 can be repeated as many times as required until the command wanted is reached
-
Use case resumes from step 3.
-
Use case 21: Undo previous command
MSS
-
User chooses to undo previous command
-
PFT restores itself to the state before the previous undoable command
Extensions:
-
1a. PFT does not find any undoable command
-
1a1. PFT informs user that there are no undoable commands executed previously
Use case ends.
-
Use case 22: Clear all entries
MSS
-
User chooses to clear all entries stored
-
PFT requests for confirmation
-
User enters confirmation
-
PFT deletes all entries
Use case ends.
Appendix E: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
9
or higher installed. -
Should respond to most commands within 2 seconds.
-
Should work on 32-bit and 64-bit environments.
-
Should be backwards compatible with data produced by the earlier versions of the system.
-
Should be able to hold up to 1000 entries without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
A user with below average to average typing speed for regular English text should be able to accomplish most of the tasks using commands as easily as using the mouse.
-
Size of program should be less than 15MBs.
-
User interface should be intuitive enough for users who are not IT-savvy.
-
User interface should be responsive based on different screen sizes.
Appendix G: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
G.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with an empty finance tracker. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
G.2. Deleting an expense
-
Deleting an expense while all expenses are listed
-
Prerequisites: List all expenses using the
listexpense v/all
command. Multiple expenses in the list. -
Test case:
deleteexpense 1
Expected: First expense is deleted from the list. Details of the deleted expense shown in the status message. Timestamp in the status bar is updated. -
Test case:
deleteexpense 0
Expected: No expense is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
deleteexpense
,deleteexpense x
(where x is an integer larger than the list size),deleteexpense n/bkt
Expected: Similar to previous.
-
G.3. Adding a budget
-
Adding a budget for a specific category
-
Prerequisite: Add a budget for food category using the
addbudget c/food $/100 ed/31-12-2020
command (if you are doing this in year 2021 or later, make sure the ed/ date is later than today’s date). Now a food budget exists in budget list. -
Test case: adding a budget for a category which already has a budget
Command:addbudget c/food $/200 ed/31-12-2020
Expected: No budget is created. Error message that budget already exists for that category shown in status message. -
Test case: adding a budget for a new category without specifying the amount allocated for that budget
Command:addbudget c/travel ed/31-12-2020
Expected: No budget is created. Error message that command format is invalid shown in status message. -
Test case: adding a budget with end date before start date
Command:addbudget c/food $/250 sd/01-01-2020 ed/30-06-2019
Expected: No budget is created. Error message for invalid end date is shown in status message.
-
-
Saving added budgets
After adding a budget, close the window then re-launch the app.
Expected: the budget list panel shows the budget(s) you had previously added before closing the window.
G.4. Editing a debt
-
Editing a debt while all debts are listed
-
Prerequisites: List all debts using the
listdebt v/all
command. Multiple debts in the list. -
Test case:
editdebt 2 $/17
Expected: Amount for second debt in the list is changed to $17. Success message shown in status message. -
Test case:
editdebt 1
Expected: First debt is not edited. Message prompting user for at least one field to be edited shown in status message. -
Also try
editdebt 0 due/31-12-2020
Expected: No debt edited. Error message for invalid command format shown in status message.
-
G.5. Adding a recurring expense
-
Adding a recurring expense to an empty list
-
Test case:
addrecurring n/daily thing $/2 c/entertainment f/d o/14
Expected: Recurring expense with specified namedaily thing
, amount$2
, categoryENTERTAINMENT
, frequencydaily
and occurrence14 times
added to recurring list with starting date set as today’s date. Success message shown in status message. -
Test case:
addrecurring n/daily sustenance $/10 c/food
oraddrecurring n/annual trip $/1500 f/y o/10
Expected: No recurring expense created. Error message for invalid command format shown in status message. -
Test case:
addrecurring n/monthly donation $/100 c/others f/m o/12 d/01-01-2019
Expected: No recurring expense created. Error message for invalid date shown in status message.
-