Overview
This portfolio aims to document the contributions made by Lim Jiahui to the Personal Finance Tracker.
Personal Finance Tracker is a desktop finance tracker application used for tracking personal finances such as daily expenses, recurring expenses such as monthly electricity bills, budgets and debts owed to another party. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 30 kLoC. The product was morphed from an Address Book over a period of 8 weeks under the constraints described here.
Summary of contributions
-
Major enhancement: added the feature that lets users manage their budgets in the finance tracker application
-
What it does: allows the user to add, edit, delete, select and clear all budgets while helping the user to keep track of how much the user has spent for each category of budget. Integrated to work with current undo/redo and history commands.
-
Justification: This feature improves the product significantly because it is now simpler for a user to track how much they are spending relative to how much they allocate to a particular category.
-
Highlights: The implementation of this feature required making significant changes to existing codes, and integrating them together. It provides a platform for any future enhancements to the finance tracker like the ability to add a recurring budget.
-
-
Code contributed: [code collated by reposense]
-
Other contributions:
-
Project management:
-
Reviewed pull requests of team mates
-
Consolidated tasks during meeting for easier reference in future
-
-
Documentation:
-
Enhancements to existing features:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Adding a budget : addbudget
Adds a budget with a time frame to the tracker.
Format: addbudget $/AMOUNT c/CATEGORY [sd/START_DATE] ed/END_DATE [r/REMARKS]
Shortcut: ab $/AMOUNT c/CATEGORY [sd/START_DATE] ed/END_DATE [r/REMARKS]
-
The categories include: FOOD, TRANSPORT, SHOPPING, WORK, UTILITIES, HEALTHCARE, ENTERTAINMENT, TRAVEL, OTHERS which are case insensitive.
-
The program only limits one budget for each category.
-
START_DATE and END_DATE must be in dd-mm-yyyy format.
-
If START_DATE is omitted, current date will be used.
Examples:
-
addbudget c/food $/400 sd/01-02-2019 ed/28-02-2019
-
ab c/others $/12000 sd/01-01-2019 ed/31-12-2019
Editing a budget: editbudget
Edits a budget in the finance tracker.
Format: editbudget c/CATEGORY [$/AMOUNT] [sd/NEW_START_DATE] [ed/NEW_END_DATE]
Shortcut: eb c/CATEGORY [$/AMOUNT] [sd/NEW_START_DATE] [ed/NEW_END_DATE]
|
Examples:
-
editbudget c/food ed/31-03-2019
-
eb c/others $/5000 sd/01-01-2019 ed/31-03-2019
Deleting a budget: deletebudget
Deletes a budget from the finance tracker.
-
Deletes the budget of the specified
CATEGORY
.
Format: deletebudget c/CATEGORY
Shortcut: db c/CATEGORY
Examples:
-
deletebudget c/food
Selecting a budget: selectbudget
Selects a budget in the finance tracker.
-
Selects the budget of the specified
CATEGORY
.
Format: selectbudget c/CATEGORY
Shortcut: sb c/CATEGORY
Examples:
-
selectbudget c/work
Clearing all budgets: clearbudget
Clears all budgets from the finance tracker.
Format: clearbudget
Shortcut: cb
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Budget Feature
This feature allows users to set budgets for different categories so they can limit the amount spent for each category.
It stores all budgets added by the user and keeps track of the expenses added within the user-specified time frame.
This feature includes addbudget
, editbudget
, deletebudget
, selectbudget
and clearbudget
.
The implementation of the budget feature is under the Logic and Model components.
Current implementation
Adding a budget:
User enters the command word addbudget
, followed by the category for which they want to set the budget for, the amount they want to limit themselves to for that category and specifies the start and end date for that budget.
Editing an existing budget:
User enters the command word editbudget
, followed by the category for which they want to edit the budget of and the relevant parameters of which they wish to edit the values of.
Deleting an existing budget:
User enters the command word deletebudget
, followed by the category for which they wish to delete the budget for.
Selecting an existing budget:
User enters the command word selectbudget
, followed by the category for which they wish to select the budget for viewing.
For all four actions, the user command gets parsed by the respective parsers: AddBudgetCommandParser
, EditBudgetCommandParser
, DeleteBudgetCommandParser
and SelectBudgetCommandParser
, which handle the execution of the commands.
Below is the UML sequence diagram of a scenario for the addbudget
command. Editing, deleting and selecting budgets work similarly to adding budgets.
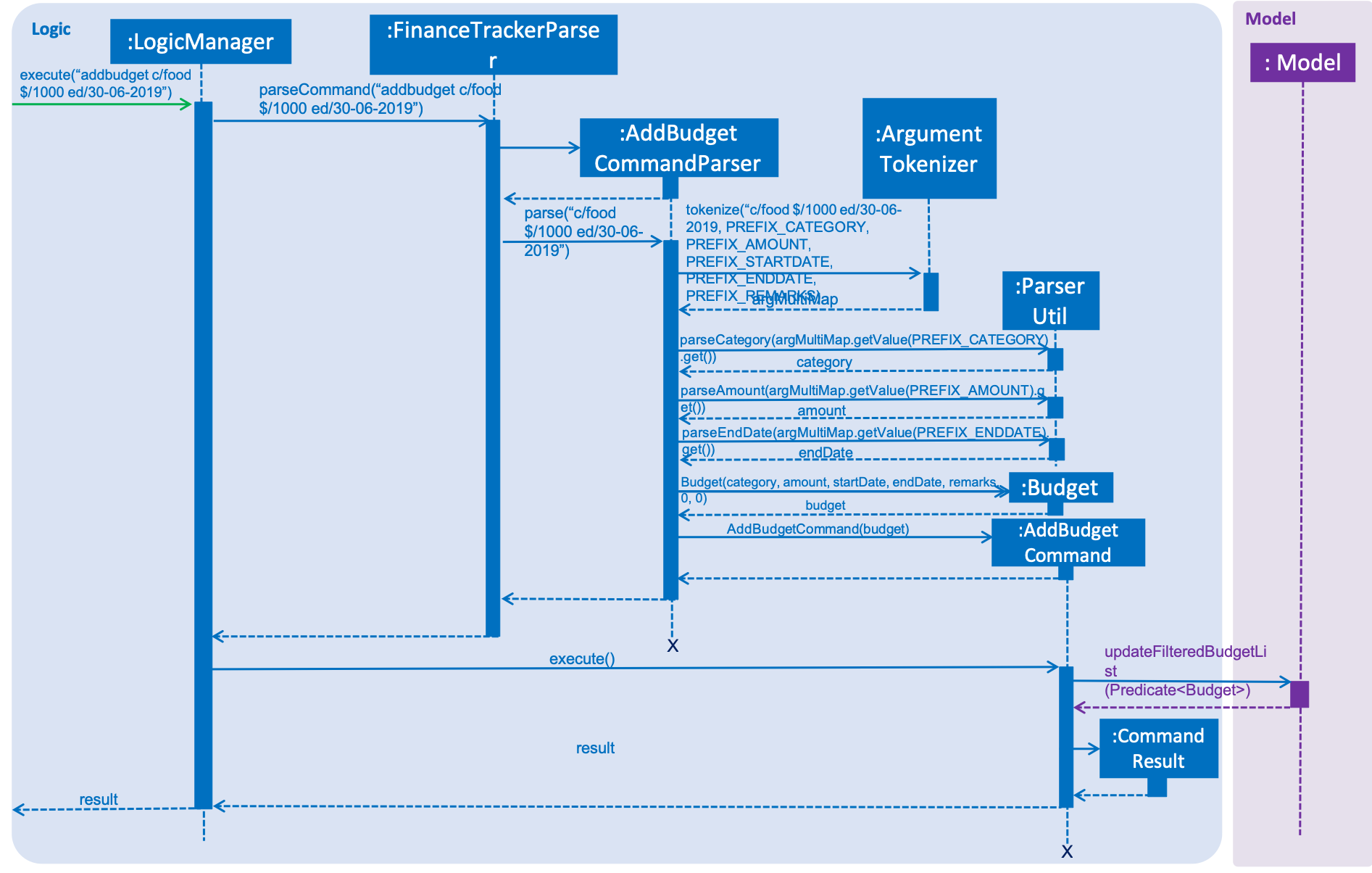
-
User enters the command
addbudget c/food $/1000 ed/30-06-2019
, to add a new budget for theFOOD
category. This command is executed byLogicManager
, which callsFinanceTrackerParser#parseCommand("addbudget c/food $/1000 ed/30-06-2019")
. This creates a newAddBudgetCommandParser
object which will help parse the input by the user. -
The newly created object calls
parse("c/food $/1000 ed/30-06-2019")
which in turn callsArgumentTokenizer#tokenize("c/food $/1000 ed/30-06-2019", "/c", "$/", "sd/", "ed/", "r/")
to split the arguments into the different parameters of a budget. This returns anargMultiMap
containing the split input. -
The
AddBudgetCommandParser
object then callsParserUtil#parseCategory(argMultiMap.getValue("c/").get())
ParserUtil#parseAmount(argMultiMap.getValue("$/").get())
andParserUtil#parseDate(argMultiMap.getValue("ed/").get())
to parse the arguments provided by the user into the correct formats of the relevant parameters. As the user does not specify a start date in this case, the programme will use the local date on the system’s clock. AnAddBudgetCommand
object containing the parameter values provided and today’s date as the start date is then created and returned to theLogicManager
. -
The
LogicManager
callsAddBudgetCommand#execute()
, which will callModel#updateFilteredBudgetList(Predicate<Budget>)
to update the list of budgets stored in the finance tracker. -
Model#addBudget(budget)
is then called to add thebudget
into the budget list. -
The command result is then returned to the
LogicManager
which then returns it to theUI
where the changes are then reflected on the user interface.
Updating of budgets:
-
When a new budget is added, the model checks the expense list for any expenses of that category which fall within the time frame of the added budget and sets the
totalSpent
andpercentage
variables of the Budget object accordingly. -
When an existing budget is edited, the model updates the
percentage
variable of the Budget object. -
For every expense the user enters, the model will check if there exists a budget for the category of the added expense and if the date of the expense is within the time frame of the budget. If the added expense is during the time of the budget, the totalSpent variable in the Budget object with that specific category will be updated.
-
The total amount spent for each budget category, along with the percentage of the total budgeted amount, will be displayed to the user so that the user can know how much has been spent so far and can better plan their future expenses so as not to exceed their budget.
Future Enhancements
The Budget class has a boolean method overlaps(Budget other)
which returns true if two budgets of the same category have overlapping time frames.
This method was included with the idea of allowing the user to add multiple budgets for the same category but for different time frames.
Another future enhancement would be to notify the user when a certain budget is about to exceed. For example, if the percentage spent within the time frame reaches say 90% of the budget amount, the user will receive an e-mail or notification of some sort to alert the user. Another notification can also be sent when the budget is actually exceeded.
Yet another possible enhancement would be to allow the user to set a recurring budget.
For example, if a user wants to limit their daily spending to $20, maybe an addrecurringbudget c/food $/20 f/d
command, similar to the recurring expense command, could be implemented so that the user does not have to add a budget everyday.
Appendix A: Product Scope
Target user profile:
-
has a need to manage a significant number of expenses, debts and bills/subscriptions
-
wants to keep track of how much is being spent
-
prefer desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: manage finances faster than a typical mouse/GUI driven app
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a/an … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use the App |
|
user |
view a list of my expenses in certain time periods |
have a better overview of my expenses |
|
user |
add expenses into the finance tracker |
record all my expenses |
|
user |
see the total amount of money I have |
see how much money I have left overall and better plan my finances |
|
user |
make changes to expenses I previously added |
correct any mistakes |
|
organised user |
categorise my expenses |
know my spending for each category |
|
user |
delete an expense |
remove entries that I no longer need to keep track of |
|
thrifty user |
set a budget |
manage my expenses and see how much more I can spend for the remaining part of the day/week/month/year |
|
user |
split my budget into different categories |
enhance my finance planning further and not spend excessively on a single category, e.g. food |
|
user |
edit my budget |
change the amount of budget available or the duration |
|
user |
cancel my budget |
|
|
user |
view a list of my debts and see when they are due |
remind myself to pay the people I owe by their due dates |
|
forgetful user |
add my debts |
can see who I owe money to |
|
user |
edit my debts |
change the amount I owe if any changes occur |
|
user |
delete my debts |
|
|
user |
clear my paid debts |
know I have settled them |
|
user |
add my recurring expenses |
keep track of my subscriptions and bills |
|
user |
edit my recurring expenses |
change the details of my bills if any changes occur |
|
user |
delete my recurring expenses |
|
|
analytical user |
see a breakdown of my expenses |
know what I spend the most on |
|
visual user |
have charts and graphs to represent my expenditure |
have a better picture on the different categories of my expenses |
|
efficient user |
be able to view my command history |
easily input a repeated command when needed |
|
user |
undo and redo my actions |
|
|
user |
clear all entries |
start with an empty finance tracker again when needed |
|
non-tech-savvy user |
type simple commands step by step |
use the programme more easily without having to keep referring to the help menu |
|
user |
receive reminders regarding my expenditure |
know if my spending is about to exceed my budget or when my debts are about to be due |
Appendix C: Use Cases
(For all use cases below, the System is the Personal Finance Tracker (PFT)
and the Actor is the user
, unless specified otherwise)
Use case 1: List expenses
MSS
-
User chooses to list all expenses and specifies if user wants to view by specific duration or category
-
PFT displays all expenses according to specified duration or category
Use case ends.
Extensions:
-
1a. PFT detects invalid value for view
-
1a1. PFT informs user that input is invalid
-
Use case ends.
-
Use case 2: Add expense
MSS
-
User chooses to add an expense
-
PFT prompts user for name of expense
-
User enters name
-
PFT prompts user for amount for expense
-
User enters amount
-
PFT prompts user for date of expense
-
User enters date
-
PFT prompts user for remark of expense
-
User enters remark
-
PFT creates expense and displays confirmation
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 10.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9)a. PFT detects wrong format or incorrect data
-
3(5,7,9)a1. PFT requests for correct format
-
3(5,7,9)a2. User enters correct data
-
Use case resumes from step 4(6,8,10)
-
Use case 3: Edit expense
MSS
-
User chooses to edit an existing expense by specifying its index
-
PFT prompts user for new name of expense
-
User enters new name
-
PFT prompts user for new amount of expense
-
User enters new amount
-
PFT prompts user for new category of expense
-
User enters new category
-
PFT prompts user for new date of expense
-
User enters new date
-
PFT prompts user for new remark for expense
-
User enters new remark
-
PFT updates the existing values to the values entered by user
Use case ends
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 12.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9,11)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11)a1. PFT requests for correct format
-
3(5,7,9,11)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12)
-
Use case 4: Delete expense
MSS
-
User chooses to delete an existing expense and specifies its index
-
PFT deletes the expense at the specified index
Use case ends.
Extensions:
-
1a. PFT detects that index is invalid.
-
1a1. PFT informs user that index is invalid.
-
Use case ends.
-
Use case 5: Add budget
MSS
-
User chooses to add a budget
-
PFT prompts user for category of budget
-
User enters category
-
PFT prompts user for amount for budget
-
User enters amount
-
PFT prompts user for start date of budget
-
User enters start date
-
PFT prompts user for end date of budget
-
User enters end date
-
PFT creates budget for specified time frame
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 10.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user that command is invalid
-
Use case ends.
-
-
3(5,7,9)a. PFT detects wrong format or incorrect data
-
3(5,7,9)a1. PFT requests for correct format
-
3(5,7,9)a2. User enters correct data
-
Use case resumes from step 4(6,8,10).
-
Use case 6: Edit budget
MSS
-
User chooses to edit an existing budget
-
PFT prompts user for category to edit budget for
-
User enters category
-
PFT prompts user for new amount for budget
-
User enters new amount
-
PFT prompts user for new start date of budget
-
User enters new start date
-
PFT prompts user for new end date of budget
-
User enters new end date
-
PFT updates the existing values to the values entered by user
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 10.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9)a. PFT detects wrong format or incorrect data
-
3(5,7,9)a1. PFT requests for correct format
-
3(5,7,9)a2. User enters correct data
-
Use case resumes from step 4(6,8,10).
-
Use case 7: Delete budget
MSS
-
User chooses to delete an existing budget and specifies its category
-
PFT deletes the budget for the specified category
Use case ends.
Extensions:
-
1a. PFT detects invalid category
-
1a1. PFT informs user that category is invalid
-
Use case ends.
-
Use case 8: List debt
MSS
-
User chooses to list debts
-
PFT lists debts
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 2
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
Use case 9: Add debt
MSS
-
User chooses to add a debt
-
PFT prompts user for expense owed for debt
-
User enters name
-
PFT prompts user for amount owed of debt
-
User enters amount
-
PFT prompts user for category of debt
-
User enters category
-
PFT prompts user for deadline of debt
-
User enters deadline
-
PFT prompts user for remark of debt
-
User enters remark
-
PFT creates budget for specified time frame
Use case ends
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 12.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9,11)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11)a1. PFT requests for correct format
-
3(5,7,9,11)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12).
-
Use case 10: Edit budget
MSS
-
User chooses to edit an existing debt
-
PFT prompts user for new expense owed for debt
-
User enters new name
-
PFT prompts user for new amount for debt
-
User enters new amount
-
PFT prompts user for new category for debt
-
User enters new category
-
PFT prompts user for new end deadline for debt
-
User enters new deadline
-
PFT prompts user for new remark of debt
-
User enters new remark
-
PFT updates the existing values to the values entered by user
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 12.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
-
3(5,7,9,11)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11)a1. PFT requests for correct format
-
3(5,7,9,11)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12).
-
Use case 11: Delete debt
MSS
-
User chooses to delete an existing debt and specifies its index
-
PFT deletes the debt at the specified index
Use case ends.
Extensions:
-
1a. PFT detects invalid index
-
1a1. PFT informs user that index is invalid
-
Use case ends.
-
Use case 12: Pay debt
MSS
-
User chooses to pay off an existing debt and specifies its index
-
PFT converts the debt into an expense entry
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 2.
-
-
1b. User enters complex command with missing parameters
-
1b1. PFT informs user of invalid command
-
Use case ends.
-
Use case 13: Add recurring expense
MSS
-
User chooses to add recurring expense
-
PFT prompts user for name of recurring expense
-
User enters name
-
PFT prompts user for amount of recurring expense
-
User enters amount
-
PFT prompts user for category of recurring expense
-
User enters category
-
PFT prompts user for frequency of recurring expense
-
User enters frequency
-
PFT prompts user for number of occurrences of recurring expense
-
User enters number of occurrences
-
PFT prompts user for start date of recurring expence
-
User enters start date
-
PFT prompts user for remark of recurring expense
-
User enters remark
-
PFT creates recurring expense and displays confirmation
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 16.
-
-
1b. User enters complex command with missing parameters
-
Use case ends.
-
-
3(5,7,9,11,13,15)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11,13,15)a1. PFT requests for correct format
-
3(5,7,9,11,13,15)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12,14,16).
-
Use case 14: Edit recurring expense
MSS
-
User chooses to edit existing recurring expense
-
PFT prompts user for new name of recurring expense
-
User enters new name
-
PFT prompts user for new amount of recurring expense
-
User enters new amount
-
PFT prompts user for new category of recurring expense
-
User enters new category
-
PFT prompts user for new frequency of recurring expense
-
User enters new frequency
-
PFT prompts user for new number of occurrences of recurring expense
-
User enters new number of occurrences
-
PFT prompts user for new start date of recurring expense
-
User enters new start date
-
PFT prompts user for new remark of recurring expense
-
User enters new remark
-
PFT updates parameters with new values
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 16.
-
-
1b. User enters complex command with missing parameters
-
Use case ends.
-
-
3(5,7,9,11,13,15)a. PFT detects wrong format or incorrect data
-
3(5,7,9,11,13,15)a1. PFT requests for correct format
-
3(5,7,9,11,13,15)a2. User enters correct data
-
Use case resumes from step 4(6,8,10,12,14,16).
-
Use case 15: Delete recurring expense
MSS
-
User chooses to delete existing recurring expense and specifies both its index and whether to delete all old expenses already added by this recurring expense
-
PFT deletes the recurring expense at the specified index
Use case ends.
Extensions:
-
1a. PFT detects invalid index.
-
1a1. PFT informs user that index is invalid
-
Use case ends.
-
-
1b. User enters command with missing or invalid parameters
-
1b1. PFT informs user of invalid input.
-
Use case ends.
-
Use case 16: View statistics summary
MSS
-
User chooses to view statistics
-
PFT prompts user for start date
-
User enters start date
-
PFT prompts user for end date
-
User enters end date
-
PFT prompts user for visual representation method
-
User enters visual representation method
-
PFT displays the statistics requested
Use case ends
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 8
-
-
1b. User enters complex command with missing parameters
-
1b1. Use case ends
-
-
3(5)a. PFT detects wrong format or incorrect data
-
3(5)a1. PFT requests for correct format
-
3(5)a2. User enters correct data
-
Use case resumes from step 4(6)
-
Use case 17: View statistics trend
MSS
-
User chooses to view statistics trend
-
PFT prompts user for start date
-
User enters start date
-
PFT prompts user for end date
-
User enters end date
-
PFT prompts user for period
-
User enters period
-
PFT displays the statistics requested
Use case ends
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 8.
-
-
1b. User enters complex command with missing parameters
-
Use case ends.
-
-
3(5,7)a. PFT detects wrong format or incorrect data
-
3(5,7)a1. PFT requests for correct format
-
3(5,7)a2. User enters correct data
-
Use case resumes from step 4(6,8).
-
Use case 18: Compare 2 statistic summaries
MSS
-
User chooses to compare statistics
-
PFT prompts user for date 1
-
User enters date
-
PFT prompts user for date 2
-
User enters date
-
PFT prompts user for period
-
User enters period
-
PFT displays the statistics requested
Use case ends.
Extensions:
-
1a. User enters complex command
-
Use case resumes from step 8.
-
-
1b. User enters complex command with missing parameters
-
Use case ends.
-
-
3(5,7)a. PFT detects wrong format or incorrect data
-
3(5,7)a1. PFT requests for correct format
-
3(5,7)a2. User enters correct data
-
Use case resumes from step 4(6,8)
-
Use case 19: View command history
MSS
-
User chooses to view command history
-
PFT lists all valid commands entered in reverse chronological order
Use case ends.
Use case 20: Auto-fill previous command
MSS
-
User keys in “Up” arrow
-
PFT auto-fills previous command stored in history in the command line
Steps 1-2 can be repeated as many times as required until the command wanted is reached
-
User presses enter
-
PFT executes autofilled command
Use case ends.
Extensions:
-
2a. User realises user has accidentally pressed "Up" arrow too many times
-
2a1. User presses "Down" arrow
-
2a2. PFT auto-fills next command stored in history in the command line
-
Steps 2a1-2a2 can be repeated as many times as required until the command wanted is reached
-
Use case resumes from step 3.
-
Use case 21: Undo previous command
MSS
-
User chooses to undo previous command
-
PFT restores itself to the state before the previous undoable command
Extensions:
-
1a. PFT does not find any undoable command
-
1a1. PFT informs user that there are no undoable commands executed previously
Use case ends.
-
Use case 22: Clear all entries
MSS
-
User chooses to clear all entries stored
-
PFT requests for confirmation
-
User enters confirmation
-
PFT deletes all entries
Use case ends.